SLC21 Week3 - Strings in C
1 comment
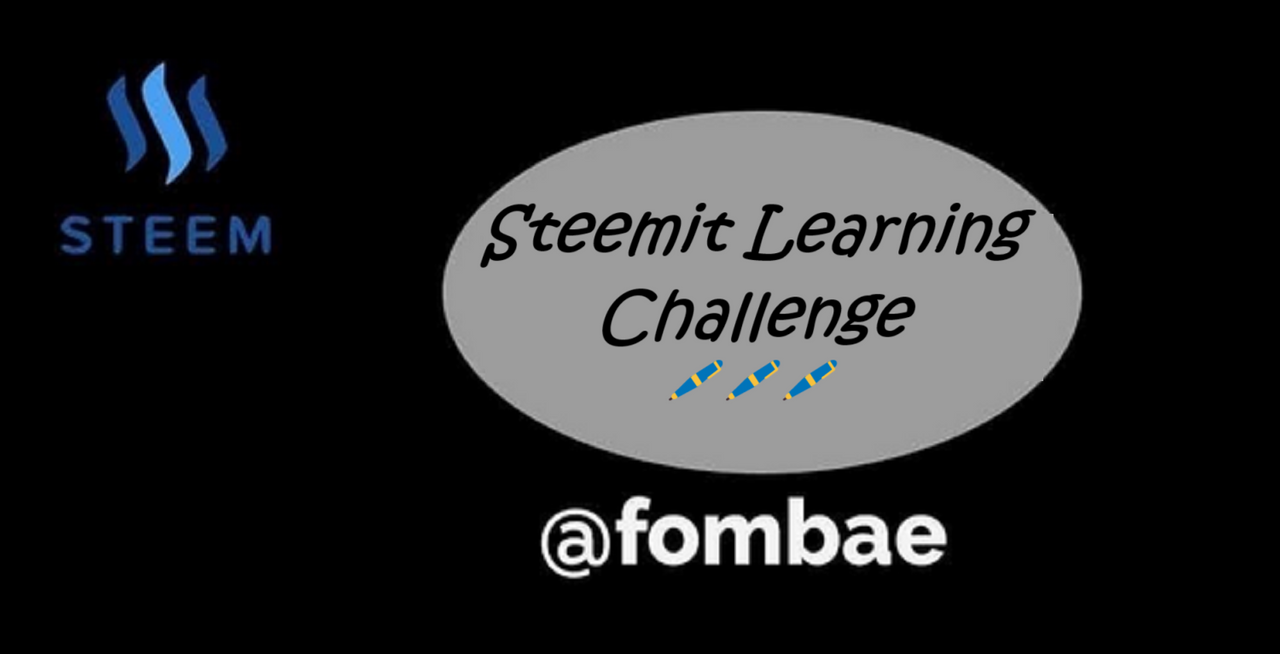
Greetings Steemit friends
Practically (i.e., with code examples) explain the theory from the first part of this lesson, where the concept of two sizes of an array is discussed. Demonstrate how to make it look like the array size can be increased/decreased. All loops should work with the array size stored in the size variable. Keep the physical, actual size in the constant N.
To be able to avoid library functions, it will be important to have a basic understanding of the concept of two sizes of an array. Our class this week is based on manipulating strings in an array, just as in the previous lesson we looked at integers. They are two sections, that is the physical and logical size of an array.
The physical size of an array refers to the number of characters that the array can hold, while the logical size is the number of characters in the array. It could be or not up to the value of the physical size.
Every array declared in this context has a physical and logical size, and if the logical size is not equivalent to the physical size. That means we have unused bytes left, which is the null terminator excluded from the physical size. For us to have the actual physical size of the array, I will define a constant N. Here I will be able to use a variable to represent the logical size of the array.
- Example
I believe from the image above, we have a better understanding of the key concepts of the two sizes of an array. In the example, my physical size was 50 as declared in the variable N. While my logical size was 14 for the string Hello! fombae.
Now let's look at how we can increase or decrease the array size. Let's start by adding characters to the array size. Note we need to be aware of the initial size, which is N [50]
. we can only add if we have more space, and in our case we have space.
50-14 = 36
From my example above I have 36 unused bytes, giving allowance to add more characters to the array. Will add the characters one after the other using a post-increment operator(length++). The \0 null terminator is used to signify the ending of the new newly added string. At this point, the logical size has changed.
Decreasing the array, we make use of the post-decrement operator(length--). This is done too one after the other, as we were adding. Here we will have to take the last character one after the other, depending on how many characters we want too.
The last section requires us to make use of the logical size and ignore the unused bytes of the physical size. I use the for loop to access each character up to the logical size.
Here we have printed each character, and can still use an if statement in the loop to manipulate (modify) each character.
Task: reverse the string
Okay for me to achieve this, I need to declare a variable to hold the string. Next, I need to figure out the logical size for the string, which I can modify the positions of the characters. How to move the characters is what we try to figure out. If we can place characters using their positions(index), then it will be easy to figure it out from there.
Declare and initialize the string "ABCDEF", and get the logical size by counting the characters in the string. I print it out to be sure I'm getting the correct size. It's okay we can take it off after getting our final result.
Now, how to reverse the string. To be able to manipulate the characters and make the positions, I used a loop. For each loop, I create a variable to hold the character using the index temp. Next, I try to swap the position by assigning a value for the index. It worked for one character and I tried to repeat the same process, but it did not work as expected.
Wrong approach.
Okay, I try working in pairs, which seems to be the best approach. Divining the logical size by 2 will lead to manipulating two chars at once. But something is still missing, as only two characters change position.
To make some changes
For the first loop
s[i] = s[length - 1 ];
that means s[0] = s[5];
Second loop
s[i] = s[length - 1 ];
that means s[1] = s[5];
last loop
s[i] = s[length - 1 ];
that means s[2] = s[5];
This is the situation above, the second and last loop have not made any changes to our string. I noticed I could subtract it to get a different position one step down.
For the first loop
s[i] = s[length - 1 - i];
=> s[0] = s[5];
Second loop
s[i] = s[length - 1 - i ];
=> s[1] = s[4];
Last loop
s[i] = s[length - 1 - i];
=> s[2] = s[3];
s[0] = A, s[5] = F
s[1] = B, s[4] = E
s[2] = C, s[3] = D
This made it easy to swap positions making use of the character store in the template.
s[length - 1 - i] = temp;
At this point, reversing characters from both ends was possible until we got to the midpoint.
Swap neighboring letters char s[]="ABCDEF";.....your code.....cout<<s; => BADCFE
For this task, I will just make some changes after getting some basics from the previous task.
Declaring and determining the logical size is the first step. Next will be swapping neighboring letters, which will mean setting the loop by an increase of two as we will be working with two characters.
First store the character at index i, next assign a +1 to index i. This will give us the index of the neighboring character for us to finalize the swap.
i = 0 => s[0] = s[1] ( A = B)
This will complete the swapping of the remaining characters in the array.
Shift the string cyclically to the left (it’s easier to start with this), then cyclically to the right. char s[]="ABCDEF", x[]="abrakadabra";.....your code.....cout<<s<<"\n"<<x; => BCDEFA aabrakadabr
Well, we will continue to modify the characters of the array. During the first task, I was able to archive shifting my position to the right. Now we try to perfect and have a much clearer result.
Just like the previous task, I Declared and determined the logical size of the string. Next, we store the first character and use the loop to shift each character by one position. Finally, replace the last character with the first.
The same step will work to shift to the right, what we will be changing will be the operation sign. To shift to the left, each for loop iterates moves an i + 1
into the position of i
. As for the right, each for loop iterates moves an i - 1
into the position of i
.
Remove all vowel letters char s[]="this is some text";...your code...cout<<s; => ths s sm txt
Just like the previous task, we have to declare and get the length to have the logical size of the array. Next, we set a new variable to handle the new position of the valid characters.
In this case, for each loop it iterates. We used the if statement to check if the character is a vowel or not. In case the condition is true (not a vowel), it is stored in the new position.
Double each vowel letter char s[]="this is some text";...your code...cout<<s; => thiis iis soomee teext
Okay, we already have somewhere to start from. From the above task, we can identify which of the letters are vowels. So in this case, we will have to check for availability and double.
I have to iterate through each character in the array when we identify a vowel. We can assign a new output to repeat the character in question.
If you try with the previous task by just changing operation && to OR and != to ==. You will not get an accurate result, and it will not be fixed. I have to assign a new array variable.
The next problem will be the new array variable. I had to add physical size, which gave the expected output. This takes us back to task one, working beyond the physical size of an array.
Cheers
Thanks for dropping by
@fombae
Comments