SLC S21 Week4 || Mastering String Manipulation and Operations in Python
1 comment
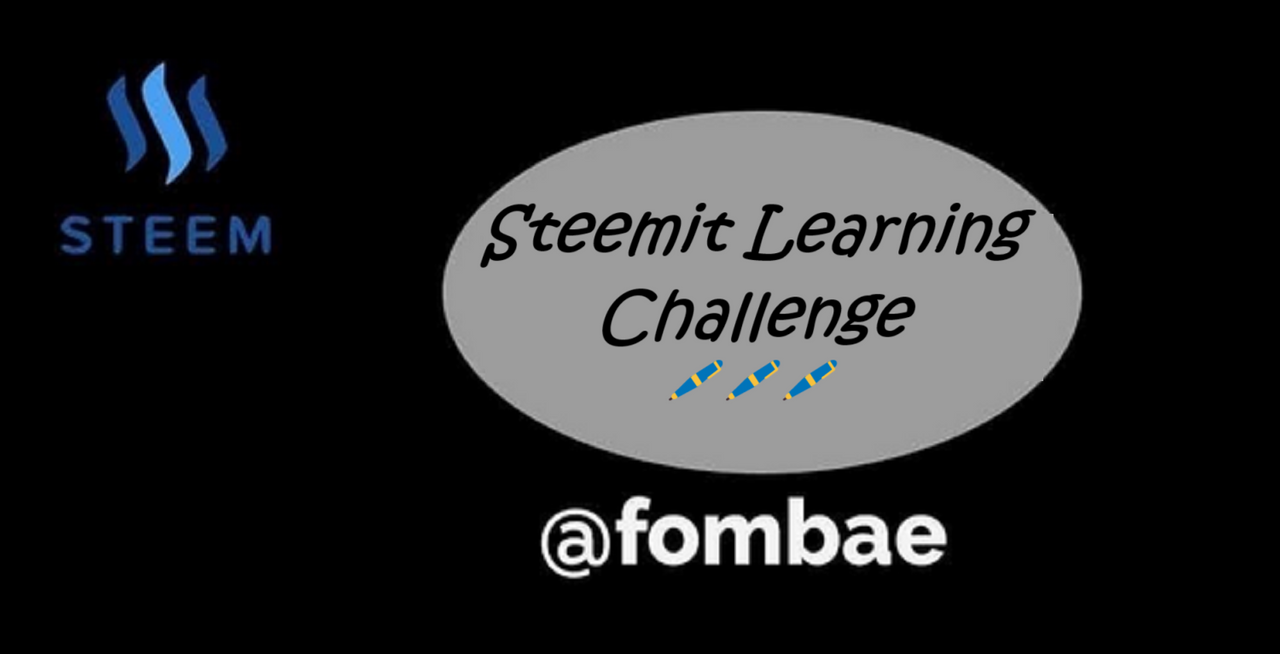
Greetings Steemit friends
Create a Python program with a graphical user interface (GUI) using PyQt5 to find the intersection of characters between two strings.
We are back in Qt Designer, to work out the graphical interface for our program. For this task, I start by launching the Qt designer application which we installed during the first week of this course. I will be making use of the widget box, that is the QLable, QlineEdit, QPushButton, and QtextEdit.
To make easy use of the object in the Thonny application, I will give QlineEdit, QPushButton, and QtextEdit unique names.
- QLineEdit widgets are used for the input section for characters 1 and 2.* QPushButton widgets are used for the button section for Form and Erase.
- The QtextEdit widget is used to display the section for the output
To have style, go down to the property section of each object and look for the stylesheet. Click the three dots in the value section and apply your desired style in the dialog box.
Now we can save our design .ui, and save it in the same direction that we will have a .py file.
Let's launch the Thonny application and move to the directory holding our .ui file. I will create my .py file. I start my application by importing the necessary modules, such as sys
to manage arguments and PyQt5.QtWidgets
to handle objects for GUI, and PyQt5.uic
to load the .ui
file.
Now I will create a function to get the two inputs and look for the intersection. Note, that our function has to check each input value, making sure it is within 1 to 30 characters and all in lowercase.
I have assigned two variables string1 and string2 to hole the values of the two inputs (input_char_1
and input_char_2
) in the .ui
file.
Now we have value, let's check if the character length is within range. That is 1 to 30, and that is for both inputs. With the AND operator, we can go ahead and check if the string islower
(lowercase)
If all inputs are valid(string1
and string2
). Loop through each character of string1 and check if any characters found in string2 have not been in string1. The characters are found, held, and displayed as a string.
The other functions are the Reset function which is to erase all the fields as demanded by the task.
2). Create a Python program with a graphical user interface (GUI) using PyQt5 to reverse the characters of each word in a string, preserving the word order.
Task 2, we are back to our QT designer to work on a graphical interface for our program. I made use of the widget box, which is the QLable, QlineEdit, QPushButton, and QtextEdit.
- The QLineEdit widget is used for the input section for strings.
- QPushButton widgets are used for the button section for text Mirror and Erase.
- The QtextEdit widget is used to display the section for the output
I will save my .ui
file and move over to the Thonny application. I created a new .py
file for the second task. A lot has not changed, I will copy the code on task 1 and paste it here. I will create the function and have the correct operation to complete the task.
- Check the range between 1 and 50, no longer 1 and 30.
- Check the spaces between characters and make sure they are all in lowercase.
- Check if there is a space at the beginning or the end of the string
- Check-in case of double spaces.
I used the if statement to validate the range, just as in task 1. to handle each character in the string, to make sure we don't have any space or the uppercase character. The all()
function is to iterate through each character in the string. Next, the startwith and endwith are used to check if the string has any space at the beginning or the ending. Finally, check if there is a space in the string.
If all the conditions return true, we can split the string, reverse it, and display it.
3). Create a Python program with a graphical user interface (GUI) using PyQt5 to encrypt a string by combining Caesar cipher rotation (by 13 positions) and reversing the rotated string.
The Qt design here is not different from the previous task. So I have just copied and pasted in the new window for the task. Note you used the same object names, or renames for your understanding. Save the .ui
file.
I moved to the Thonny application and created my .py
file. In the function, this task requires just two checks.
- Character length between 1 and 9
- Character inputs are lowercase.
Now rotate the characters by 13. we convert the characters by iterating each in the string to assign an index starting from a = 0
. Next, we wrap the index within the range 0 to 25, equivalent to the 26 alphabetical later.
When you get the index of the character in the string, add 13 and divide by 26. The remainder gives us the index of the encrypted character. The join() is used to join the characters to a single string to be rotated.
4). Create a Python program with a graphical user interface (GUI) using PyQt5 to verify whether two positive integers form a "perfect succession."
I'm sure we all have the basics of designing our graphical interface. So I will look at what is expected to have our program running smoothly. To have a valid string, all inputs need to be digits and positive integers.
To have the succession, we need to combine the two inputs to form a single string and sort. That is arranged in an incremental order.
Next, to verify the succession. We take each digit (character), add 1, and compare it to the previous digit. If they are equal, then our succession gradually builds up. The point we have is different, then the sequences are not a perfect succession.
Finally, use join()
to put back the digits to a single and display the result.
5). Create a Python program with a graphical user interface (GUI) using PyQt5 to process a sentence by sorting its words based on their lengths in ascending order.
Here we have a good number of checks to have a valid string to sort.
- Check if the input Is not empty.
- Check if the input Does not contain double spaces in between words.
- Check if the input Ends with a period.
- Check if the input Starts with a letter.
- Check if the input is more than 15 words.
If all the conditions return true, we have a valid string to manipulate.
To get the length of each of the words, we take off the last character which is the dot. Next, break the string into a list of words with the help of the space between them. Sort by length and use join()
to bring the words together while manually adding the space and the dot.
Cheers
Thanks for dropping by
@fombae
Comments