SLC S22 Week1 || Getting Started with Java and Eclipse
3 comments
Hello Everyone
I'm AhsanSharif From Pakistan
Greetings to you all, hope you all are well and enjoying a happy moment of life with steem. I'm also good Alhamdulillah. |
---|
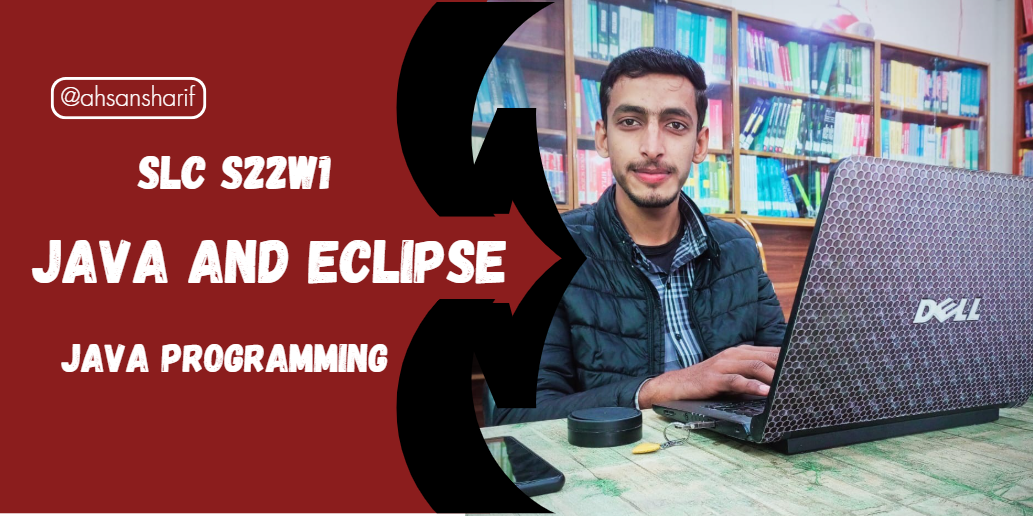
Design On Canva

Task 01

Write a detailed explanation of the differences between JDK, JRE, and JVM by including their roles, functionalities, and interdependence. |
---|
There are three important components that play an important role in Java programming, its development, implementation, and runtime. These three important components are JDK, JRE, and JVM. All of them have their own functionalities but still, they work together and enable the writing and execution of Java programs. Their details are given below.
JDK:
Role: JDK stands for Java Development Kit. It is a software development kit that developers use to write and compile applications.
Functions:
- It includes development tools such as compilers, debuggers, and utilities that are essential for developing Java applications.
- The JDK consists of the Java Runtime Environment, which is what we need to run applications, which are Java applications.
- JDK enables developers to write code and then compile it into bytecode.
- It provides us with various types of development tools that are not found in JRE. These tools include jar archiver, compiler, etc.
JRE:
Role: JRE stands for Java Runtime Environment. JRE provides us with an environment where we can execute Java programs.
Functions:
- It provides us with a runtime environment to run Java applications in which we can easily run our Java applications. It does not include any development tools etc. or compilers but rather it includes libraries which is the Java class library.
- The JRE also includes the JVM to execute Java bytecode.
- It is used to run applications that have already been compiled into bytecode. This is usually done using JDK.
JVM
Role: JVM stands for Java Virtual Machine. It acts as an abstract computing machine that enables Java applications to run on any other platform without any modification.
Functions:
- The JVM receives the bytecode and then compiles it into machine code.
- The JVM allows Java applications to run on any platform.
- It manages system resources, such as garbage collection, and then performs error handling at runtime.
- We don't need to recompile our Java programs for every other platform because different JVMs are included for different operating systems.
Interdependence:
All of them depend on each other as JDK consists of JRE and JRE consists of JVM. In this way JVM allows us to run the compiled Java code, this code is in bytecode and JRE provides us with a runtime environment and JDK provides various tools to create code.
Graphical Representation:
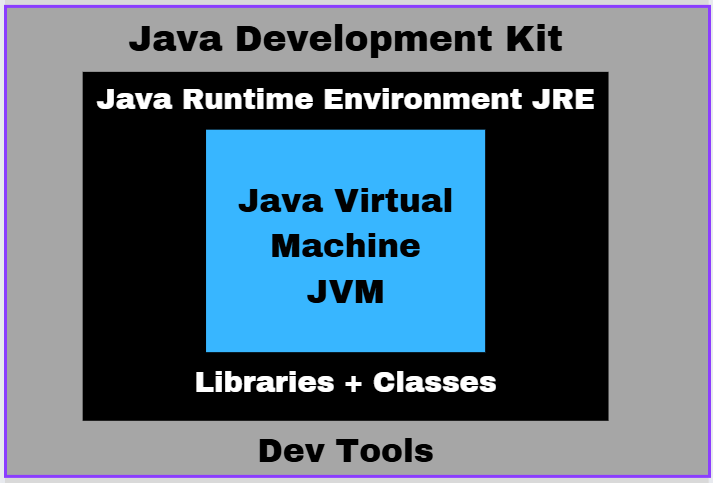
Design On Canva

Task 02

Install and Set Up Your Java Development Environment and provide step-by-step screenshots of the installation process, Eclipse configuration. |
---|
Install JDK:
To install the JDK, we will go to their official website, and from there we will install it. There are three types of links given here: Linux, Mac OS, and Windows. We are using Windows, so we will select it and from here we will select the 64-bit installer.
When it is installed with us, we will go there with the help of our PC or from our downloaded files and double-click on it, then it will start installing, so as soon as it starts, we will click on the Next button and then a path will appear in front of us, here we have to save it and after setting its path, we have to press the Next button.
This will start the installation for us. As soon as it is installed, we have to close it.
To add the Java environment, I opened my PC and after opening it, right-clicked and went to its properties. After going to properties, I opened the advanced system settings from here.
If we click on the place where Environment Variable is written, we will have an interface like the one shown below. From here we have to click on New.
From here, we have to copy and paste the name of our variable and its path so that there is no mistake and add it, thus our variable will be added.
To confirm our installation, we have to open the CMD command and confirm it by typing the Java -version
there.
Install Eclipse IDE:
To install Eclipse IDE, first, we will go to their official website and click on the download button at the top of the official website.
Then we will have different versions. We are using the Windows version, so we will click on the Windows version and download it.
After downloading, when we install it, we will have different options, out of which we have to choose the first option. As soon as we choose it, then we will have a new interface open, from there we have to install it after setting its path.
Then after that, we have to proceed with it by agreeing to all its terms and conditions and after some time we will have the installation here. After the installation, its final launch button will open where options, like Create Desktop Shortcut, will appear, we have to select them and launch them.
As soon as we click on the launch button, we will have a directory path. After setting that, we have to launch it, and finally, our Eclipse IDE is installed.

Task 03

Write a program that calculates and displays the sum of the first 100 integers. Define the main method in a class named Sum to handle the entire calculation. |
---|
We need a program that sums up our first hundred integers and displays them. Those programs are given below.
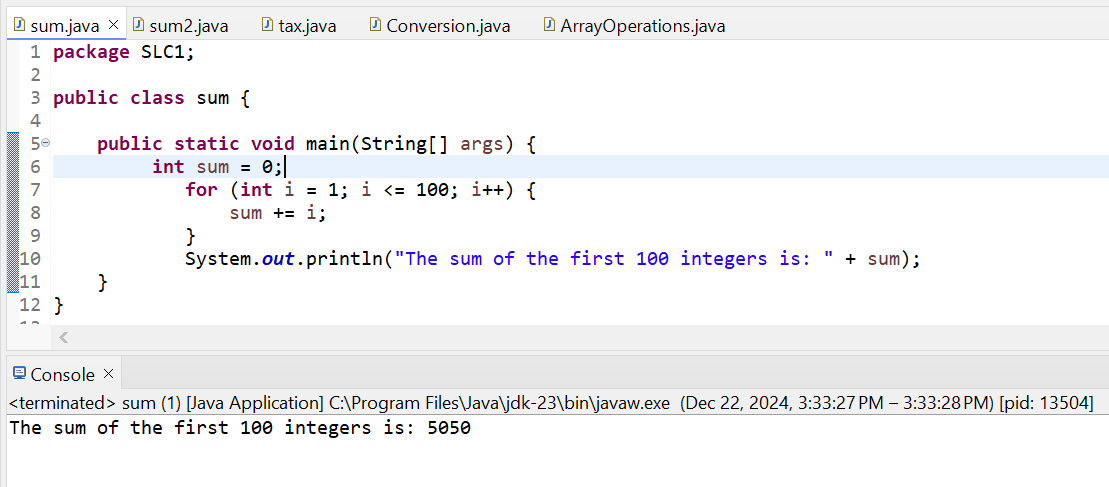
In this program, you can see that all our calculations are done using the main method. We have run our program and summed our 100 integers through the main method. We have used a loop that will run from one to 100 and each time it will add iterate to the sum. When all the loops are finished, then its sum, which is the total sum, will be printed.
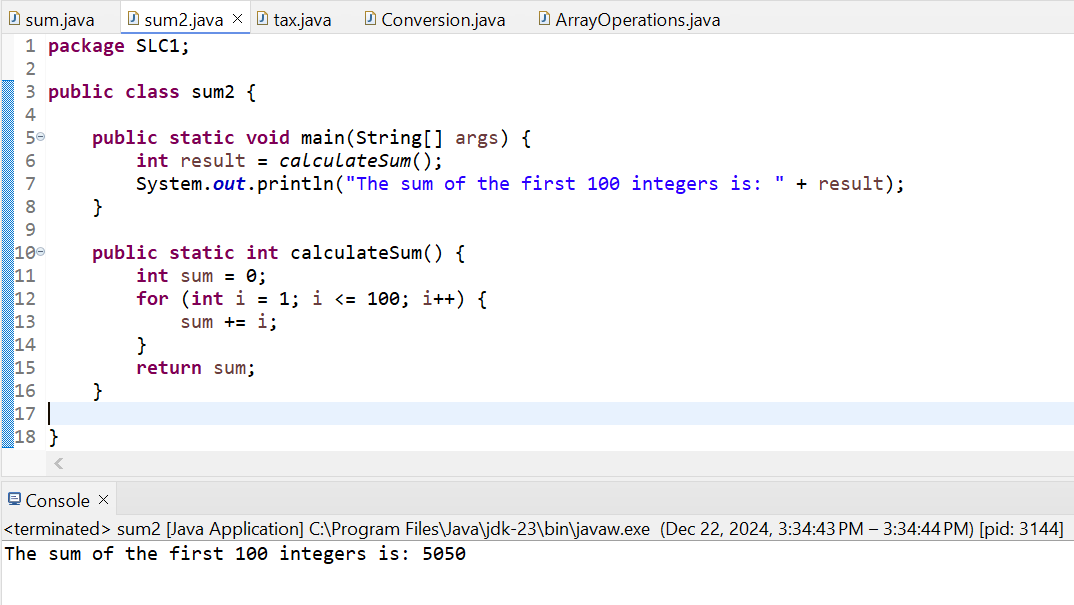
This is also similar to the first program, in that the main method will call another function called CalculateSum, which will store the value in it, sum it, and then display the result.
We have done the first program in the main method and it is a small task, we can use it for small work, but we have calculated the second program separately, this makes our code modular and reusable and we can easily maintain and extend it in the future.

Task 04

Translate, Complete, and Debug the Following Program Named Tax.java: |
---|
Here is a fully debugged version and it will calculate our text according to our given brackets and tax rate.
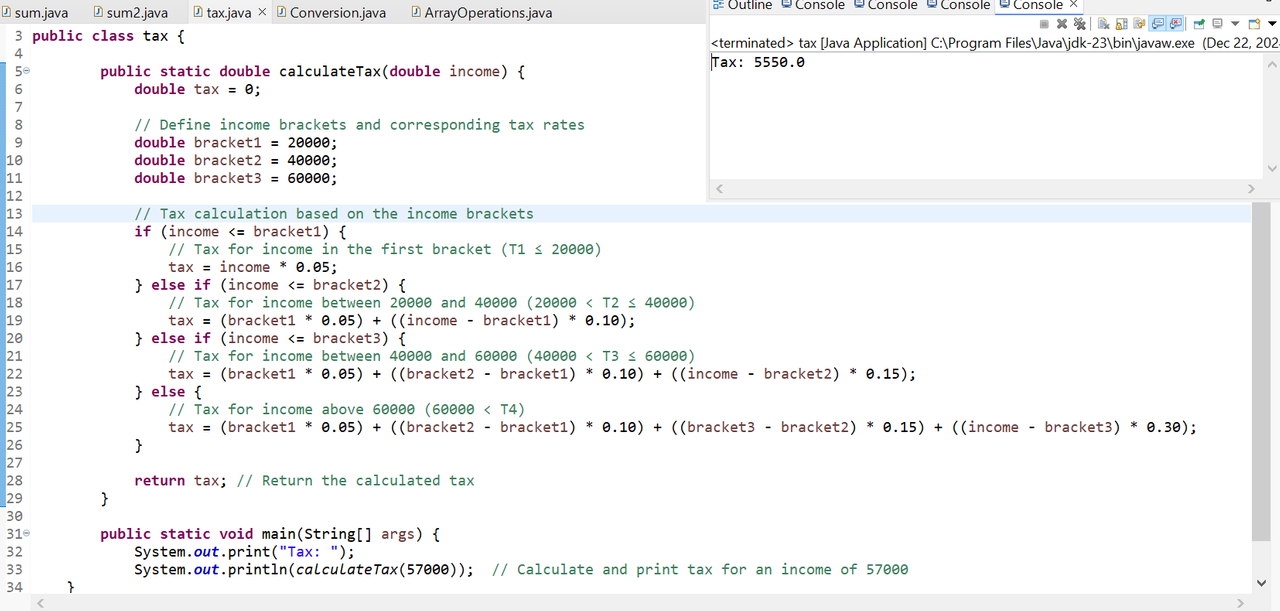
This Java program will calculate our income according to the predefined text brackets we have. For this, the CalculateText method will take our income as an input. After taking the input, it will then apply it according to the different tax rates that we have for the four income brackets. This program will calculate the tax for an income of 57,000, which is for each bracket, in which 5 percent is for the first 20,000, 10 percent is for the next 20,000, and 15 percent is for the remainder, and as a result, it will show the total tax.

Task 05

Using the same program structure as in the previous exercise, write a program named Conversion.java that takes a character c as a parameter |
---|
Here we will create a program called Conversation.java. Here our program will work, convert upper case to lower case, convert lower case to upper case and if there is no letter, it will show it.
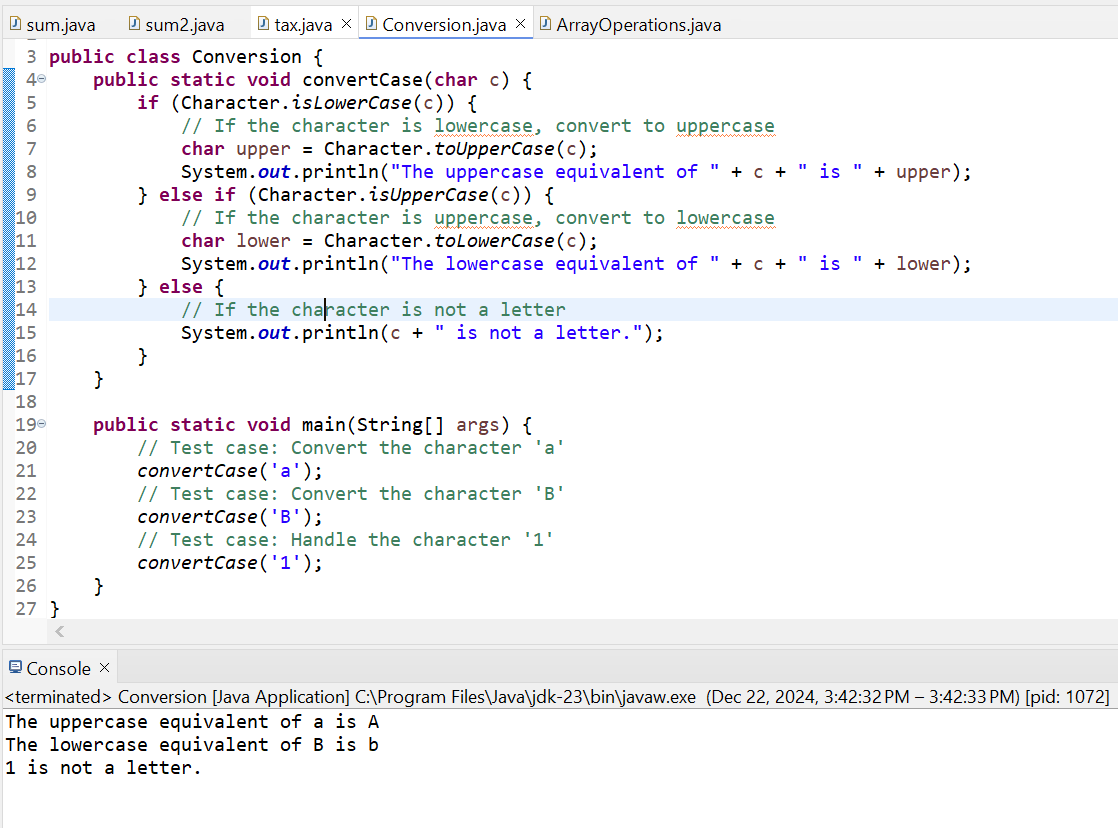
In the above program, the method Convertcase has been used and it will take the characters as input and then see whether it is a lowercase or an uppercase letter. If the character is upper case, it will convert it to lower case using the character.toLowerCase. Similarly, if the character is lowercase, it will convert it to uppercase using the Character.toUpperCase and convert it and display it. To demonstrate the functionality, we will have the main method provide characters as a sample, such as 1.

Task 06

Using a similar structure as in the previous exercises, write a program named ArrayOperations.java that performs operations on an integer array. |
---|
We need a program that performs various operations, such as finding the largest number in an array or returning the sum of the entire array and sorting it in ascending order.
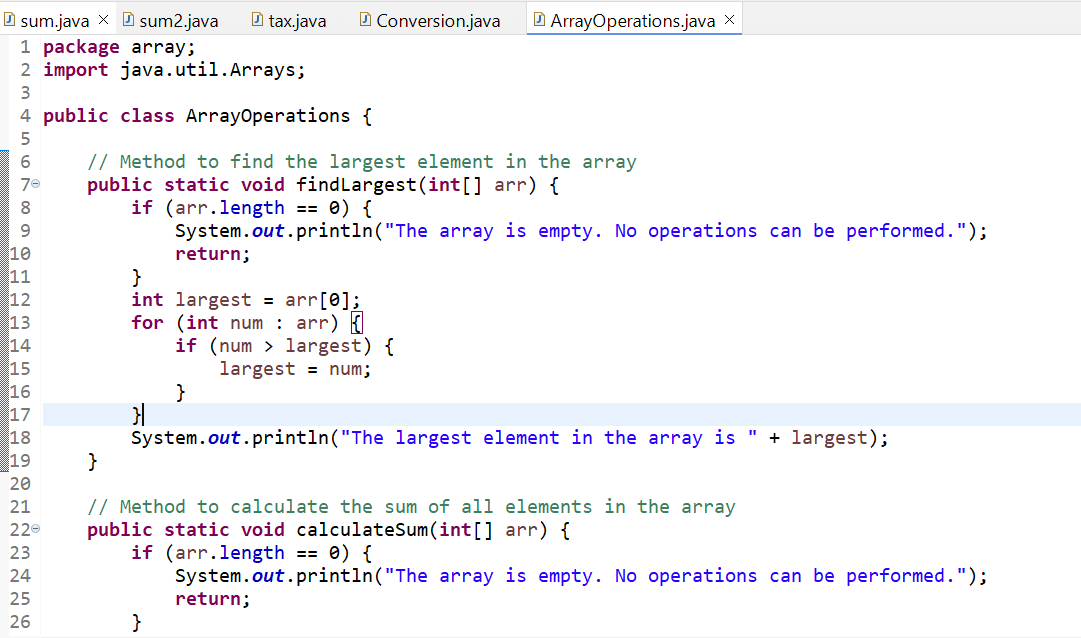
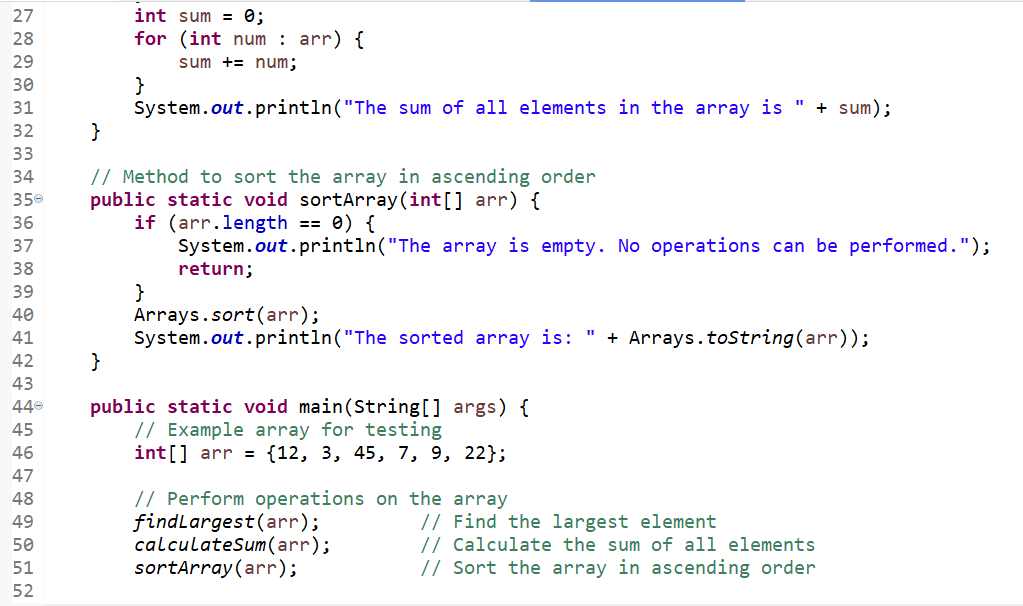
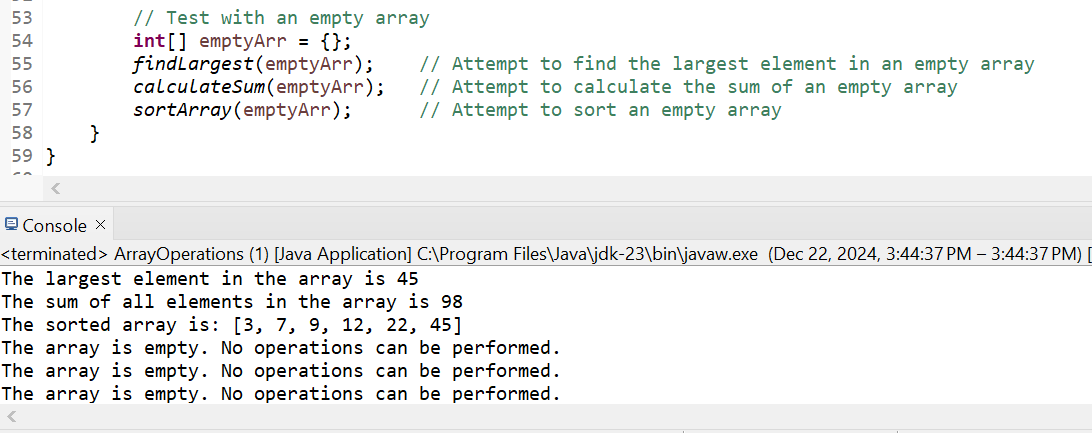
There are three main operations in this program, each operation has its implementation method. The FindLargest method loop will be used to find the largest element in this array and then the CalculateSum method will be used to calculate it. And then using the ArraySort method, we will display our array in ascending order. If the array is empty, then the program will check it and display a message. No operation can be performed.
Thank you so much for staying here guys. I would like to invite @chant, @aviral123, and @abdullaw2 to join this contest.
Cc:
@kouba01
Comments