SLC S21 Week3 || Mastering Records and Record Arrays with Python
1 comment
Assalamualaikum my fellows I hope you will be fine by the grace of Allah. Today I am going to participate in the steemit learning challenge season 21 week 3 by @kouba01 under the umbrella of steemit team. It is about Mastering Records and Record Arrays with Python. Let us start exploring this week's teaching course.
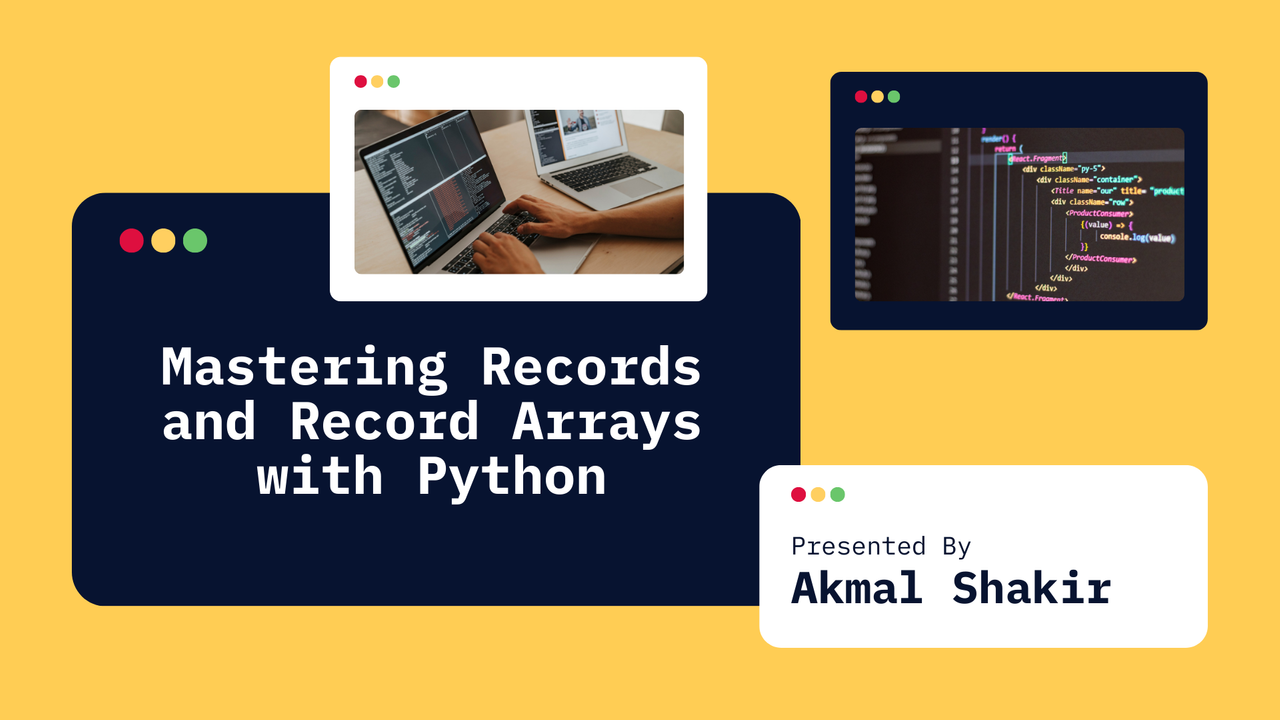
Develop a Python program to manage employee records using dataclass. The program should support adding, updating, and displaying employee information, along with calculating average performance scores and identifying top performers.
Here’s an explanation of the employee management program which uses Python's dataclass
to structure employee information and functions to manage records interactively. Each part of the code is organized to handle specific tasks such as adding, updating, and displaying employee data.
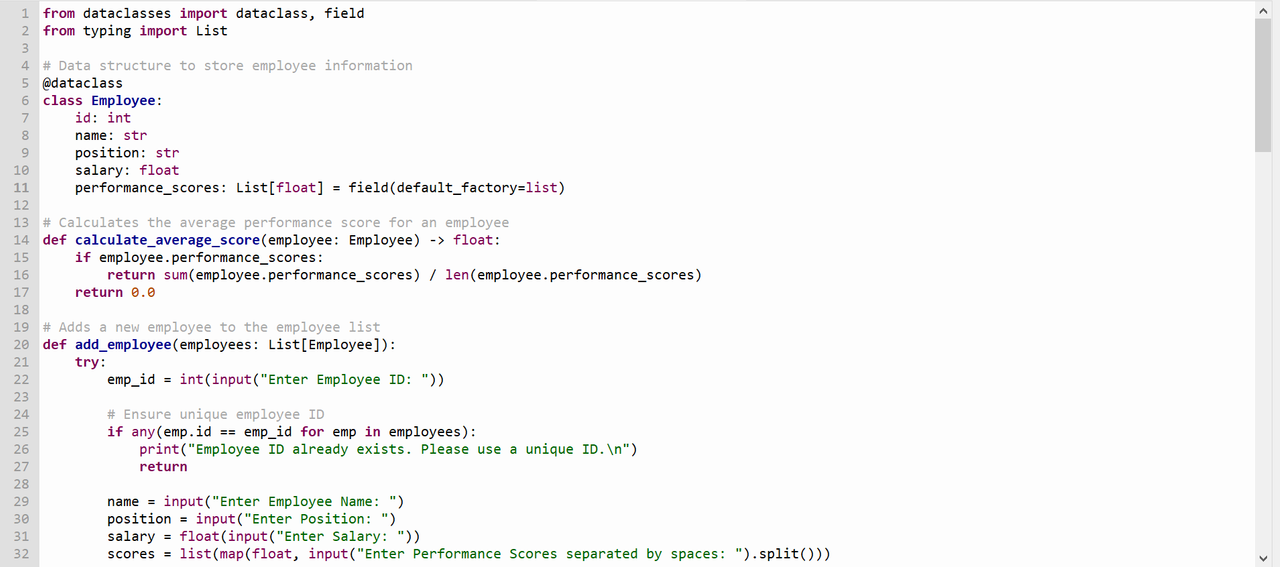
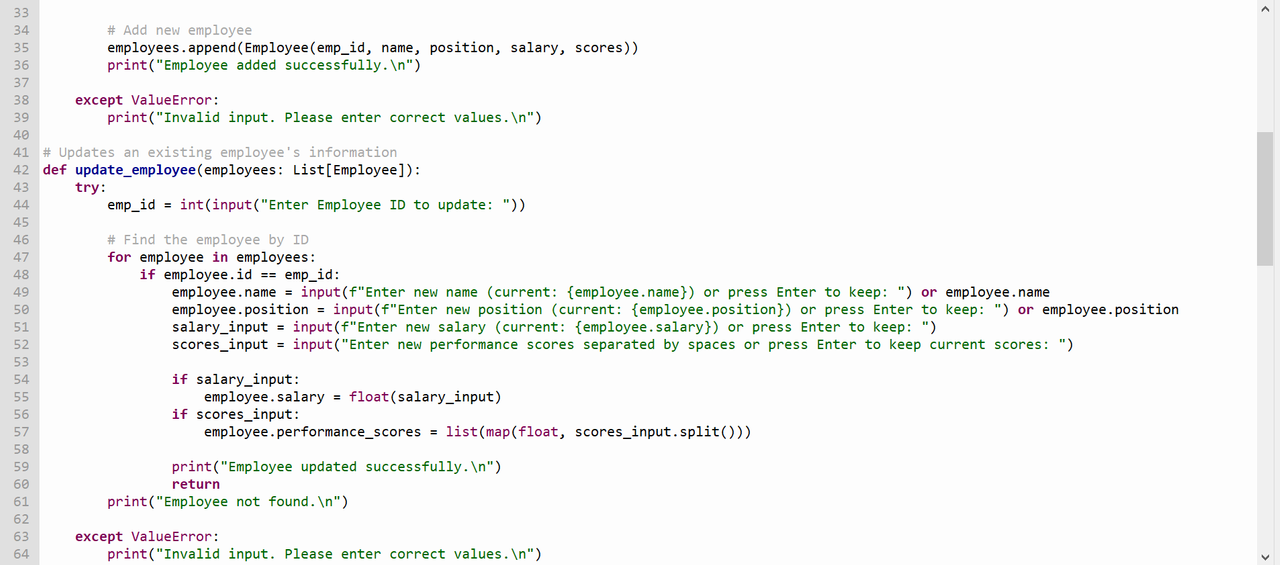
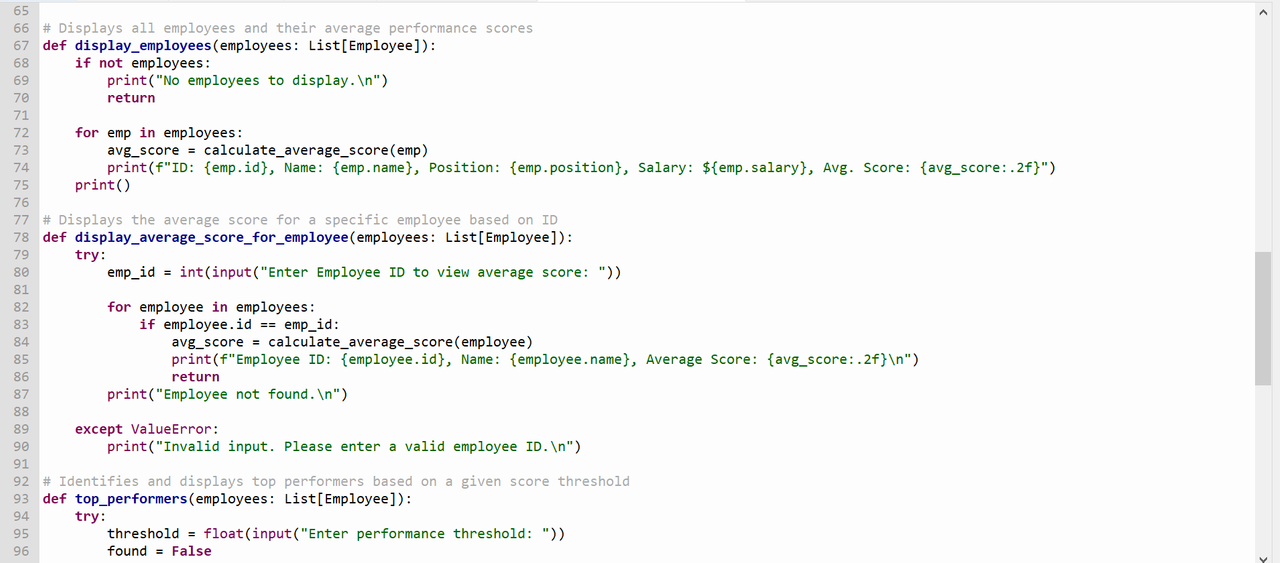
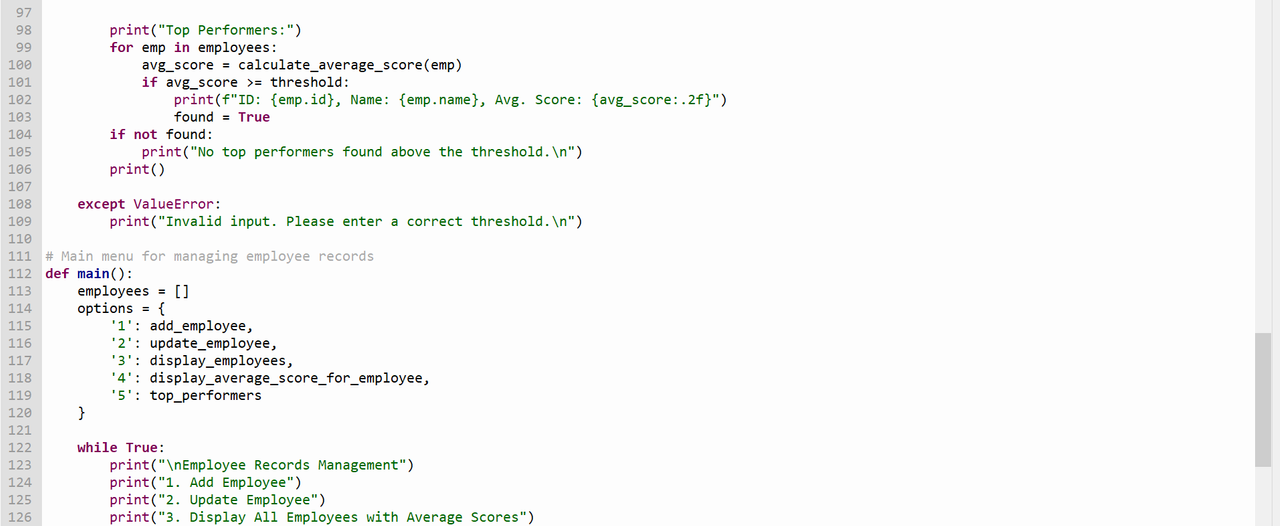
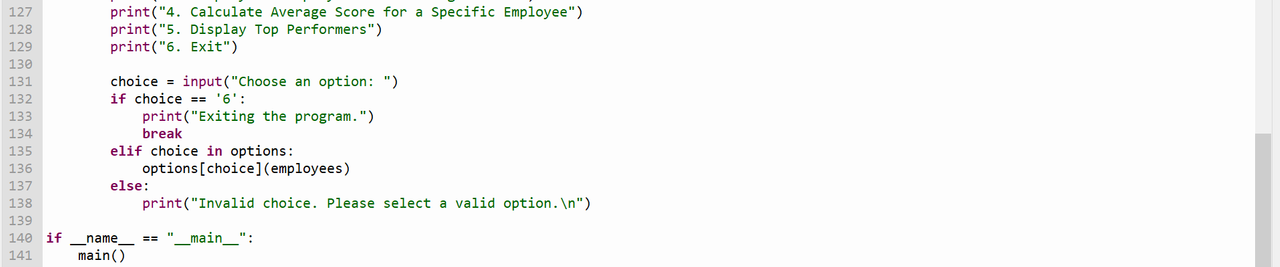
Code Breakdown
1. Imports and Setup:

dataclasses
: Simplifies creating data classes with minimal boilerplate code.field
: Allows initializing mutable types (like lists) with default values.List
: Type hint for lists used in employee records.
2. Employee Dataclass:
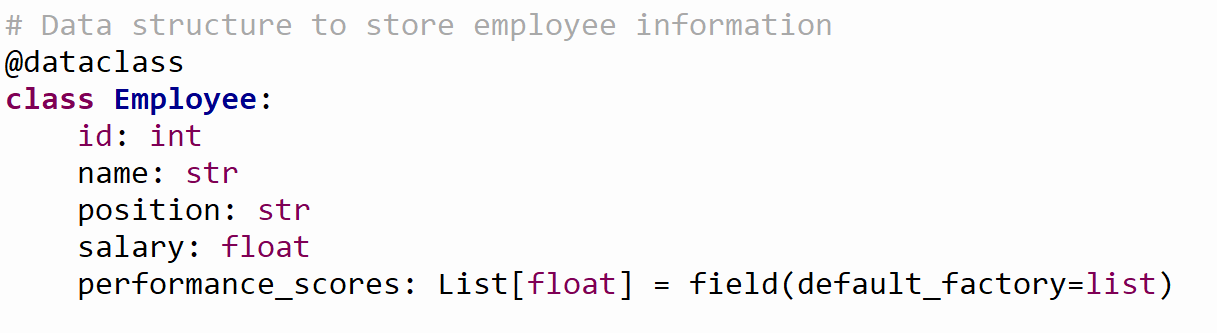
- Defines
Employee
, a simple structure holding essential employee attributes:id
,name
,position
,salary
, and a list ofperformance_scores
. field(default_factory=list)
ensures eachEmployee
instance gets a unique list for scores.
3. Function to Calculate Average Performance Score:

- Computes the average performance score of an employee.
- If the employee has no scores, it returns
0.0
.
4. Function to Add a New Employee:
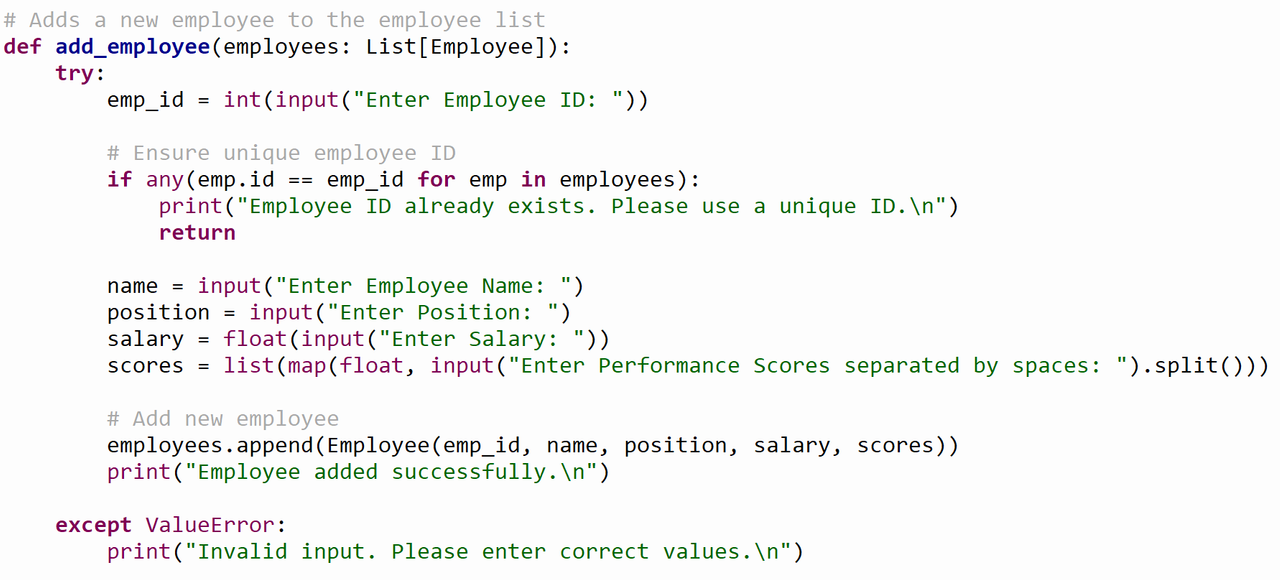
- Prompts the user to enter details like
ID
,name
,position
,salary
, and performance scores. - Checks for unique employee IDs to avoid duplicates.
- After gathering information, it creates a new
Employee
instance and adds it to the list.
5. Function to Update an Existing Employee’s Information:
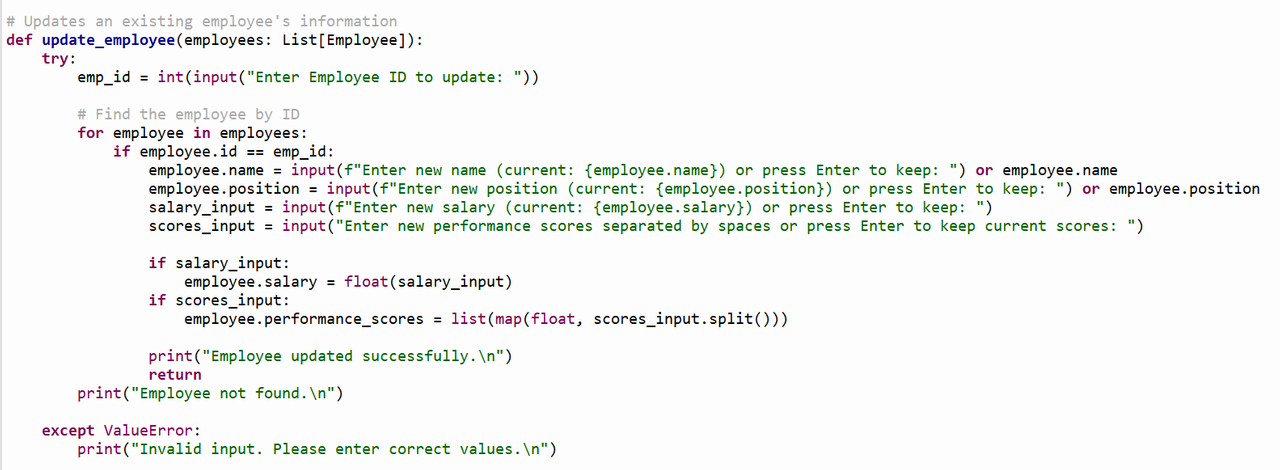
- Prompts the user for the employee’s
ID
to locate the record. - Asks for updated values for each attribute and provides options to skip any updates (by pressing Enter).
- Converts inputs as needed, and only updates fields where new values are provided.
6. Function to Display All Employees with Average Scores:

- Loops through the
employees
list and prints each employee's details. - Calls
calculate_average_score()
to display each employee's average performance score. - Displays a message if there are no employees to show.
7. Function to Display Average Score for a Specific Employee:
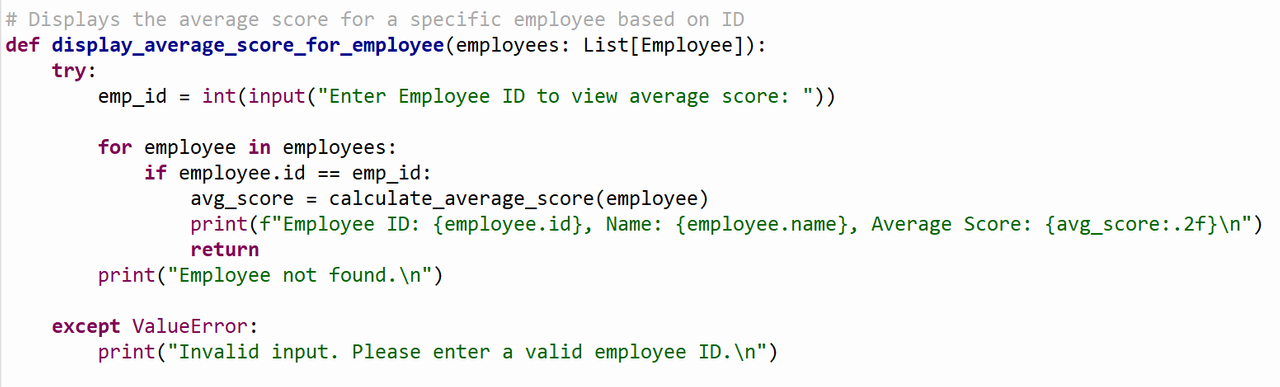
- Asks for an employee’s
ID
to calculate and display their average performance score. - If the employee ID is found, it prints the average score; otherwise, it notifies that the employee was not found.
8. Function to Identify Top Performers:
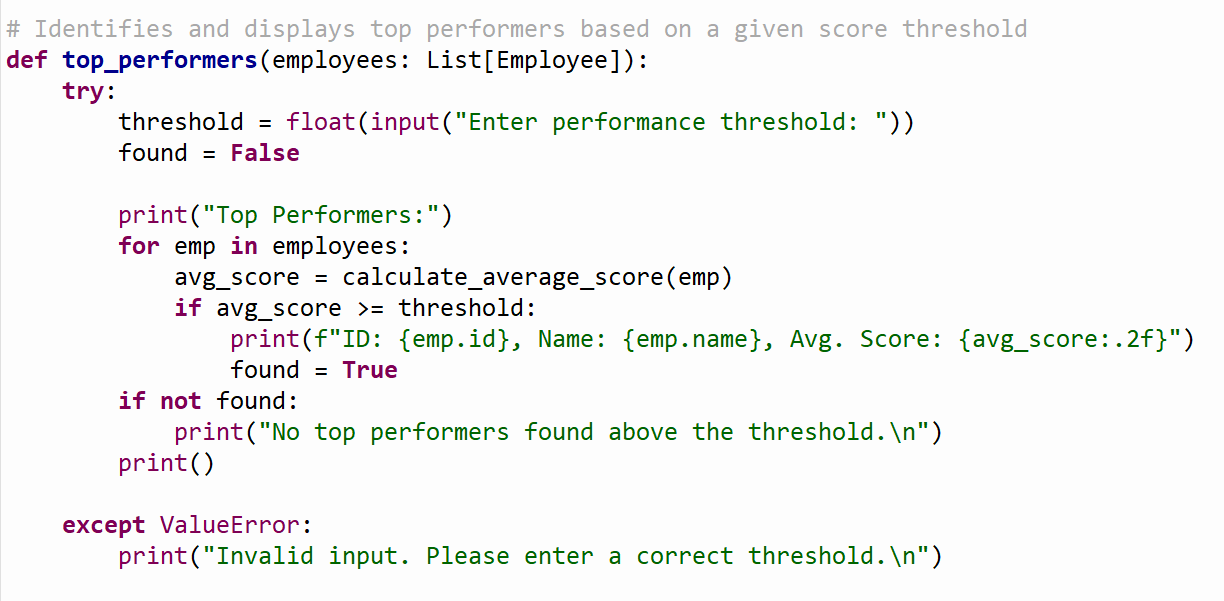
- Accepts a performance threshold from the user.
- Prints details of employees whose average score is equal to or exceeds this threshold.
- If no employees meet the threshold, it displays a relevant message.
9. Main Function to Manage the Interactive Shell:
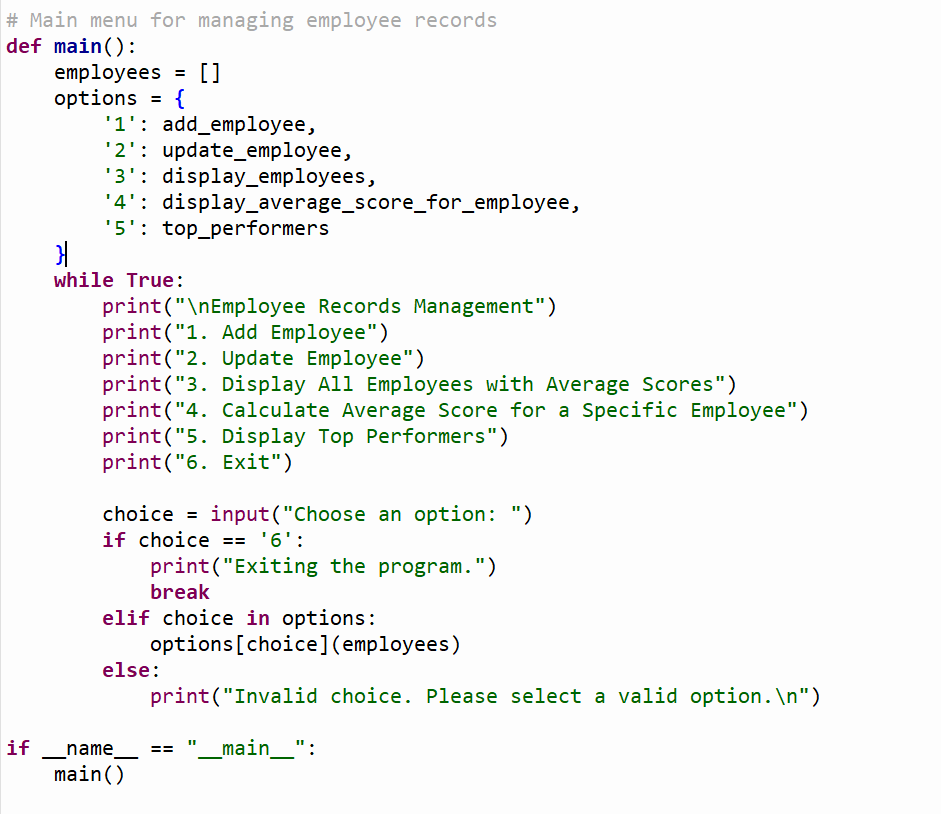
- Provides an interactive menu for the user to select options like adding or updating employees, displaying records, calculating scores, and identifying top performers.
- Uses a dictionary (
options
) to map menu choices to respective functions, making the code modular and readable. - The program continues looping until the user chooses to exit (
6
).
This program provides a straightforward, interactive way to manage employee records. It is ideal for learning about structured data handling in Python.
Utilize NumPy's structured arrays to store and analyze student grades, including calculating averages, identifying top students, and analyzing subject-wise performance
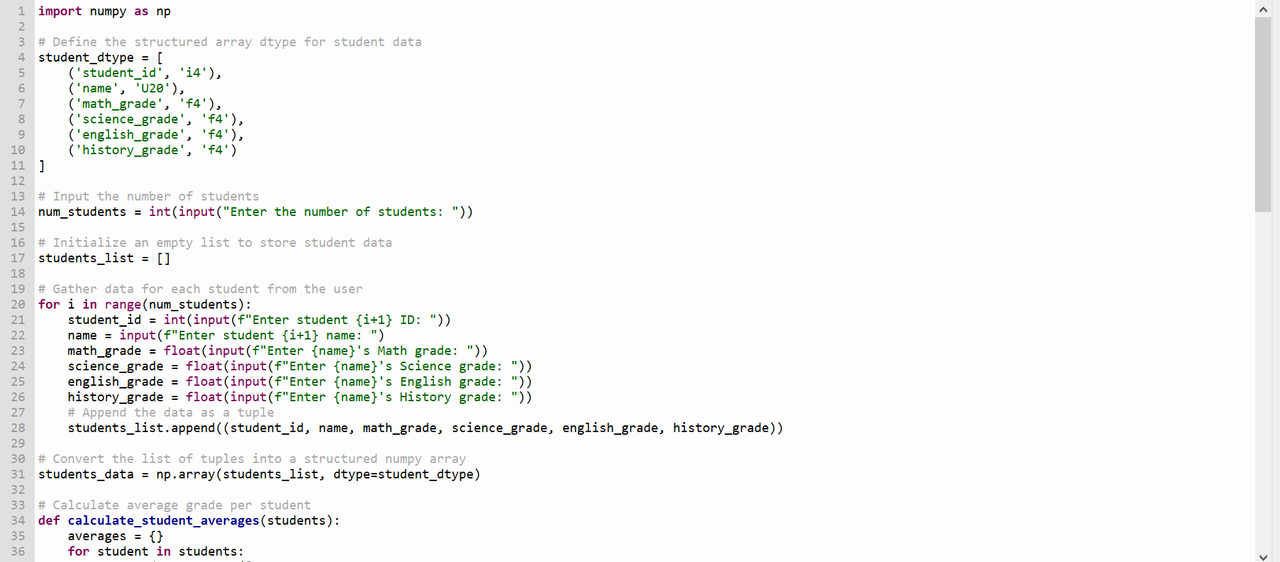
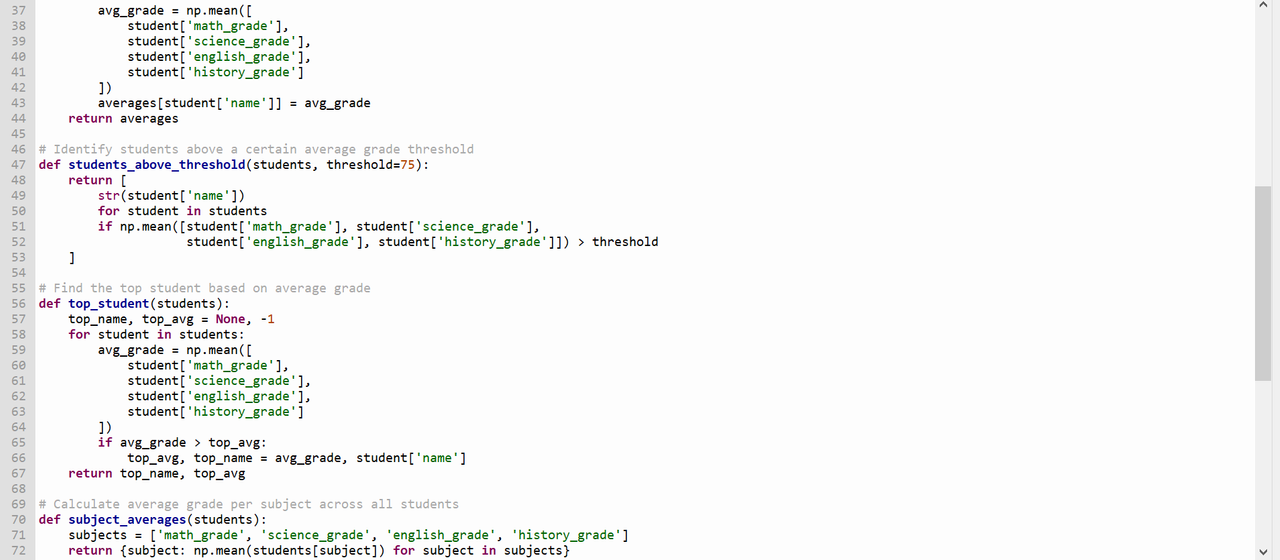
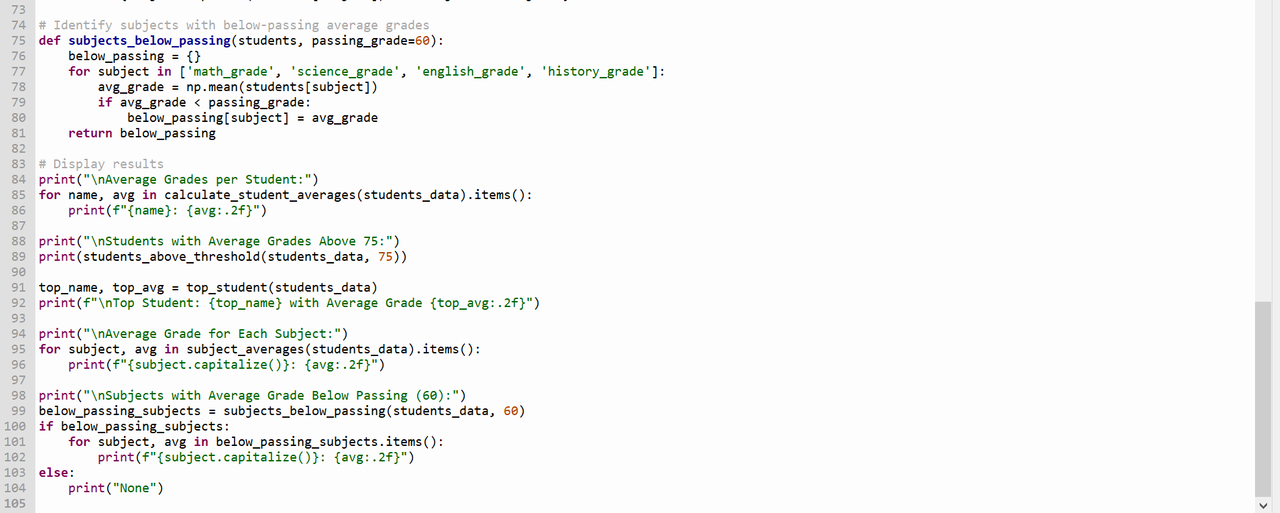
Code Breakdown
Here is an explanation of the code:
First of all I have imported NumPy
library to perform the mathematical computations.
1. Define the Structured Array dtype
for Student Data:
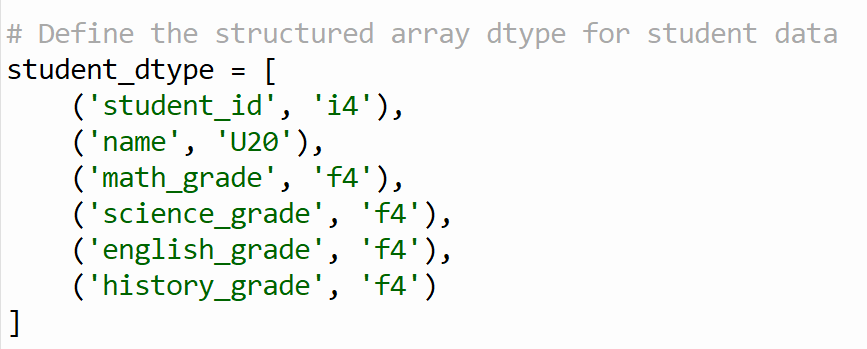
student_dtype
defines the structure of the student data using NumPy's structured array type. This ensures that the data is organized in a format with fields likestudent_id
,name
, and grades for different subjects (math_grade
,science_grade
, etc.).'i4'
refers to a 4-byte integer for the student ID.'U20'
is a string of up to 20 characters for the student's name.'f4'
is a 4-byte float for the student's grades.
2. Input the Number of Students:

- This line asks the user for the number of students they want to enter data for, and stores it in
num_students
. The input is converted to an integer usingint()
.
3. Initialize an Empty List to Store Student Data:

- An empty list,
students_list
, is initialized. This list will later hold each student's data as tuples.
4. Loop to Get Data for Each Student:
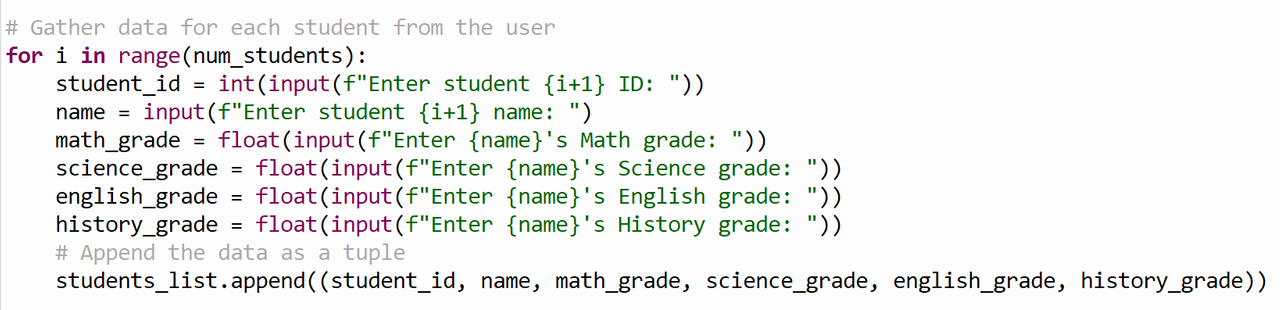
- This loop runs
num_students
times, asking the user to enter details for each student:- Student ID is entered as an integer.
- Student Name is entered as a string.
- Grades for each subject are entered as floats.
- After gathering the data for a student, it is appended to the
students_list
as a tuple.
5. Convert the List to a Structured NumPy Array:

- After all the student data is collected in the list, the list is converted to a structured NumPy array called
students_data
using thenp.array()
function. Thedtype=student_dtype
ensures that the array follows the structure defined earlier.
6. Calculate Average Grade Per Student:
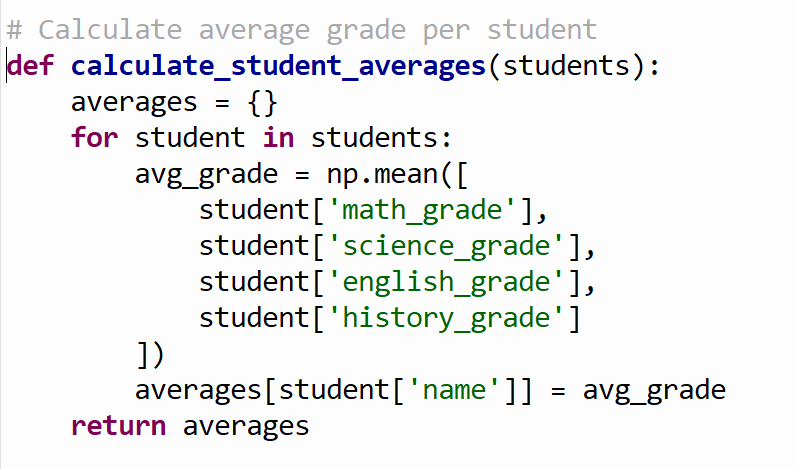
- This function calculates the average grade for each student by:
- Iterating through the
students
array. - Calculating the mean of the four subject grades for each student using
np.mean()
. - Storing the student's name and their average grade in the
averages
dictionary. - Finally, it returns the dictionary with the average grades.
- Iterating through the
7. Identify Students Above a Certain Grade Threshold:
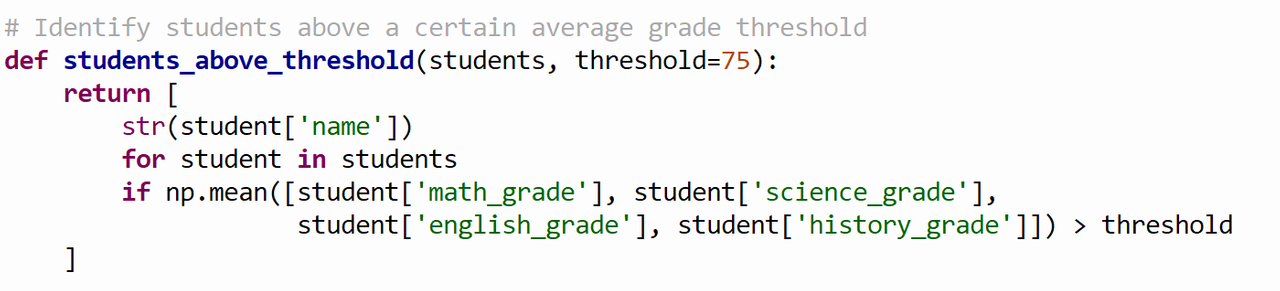
- This function identifies students whose average grade exceeds a specified threshold (default is 75):
- It iterates through the
students
array. - For each student, it calculates their average grade.
- If the average is greater than the
threshold
, the student's name is added to the list, which is returned at the end.
- It iterates through the
8. Find the Top Student Based on Average Grade:
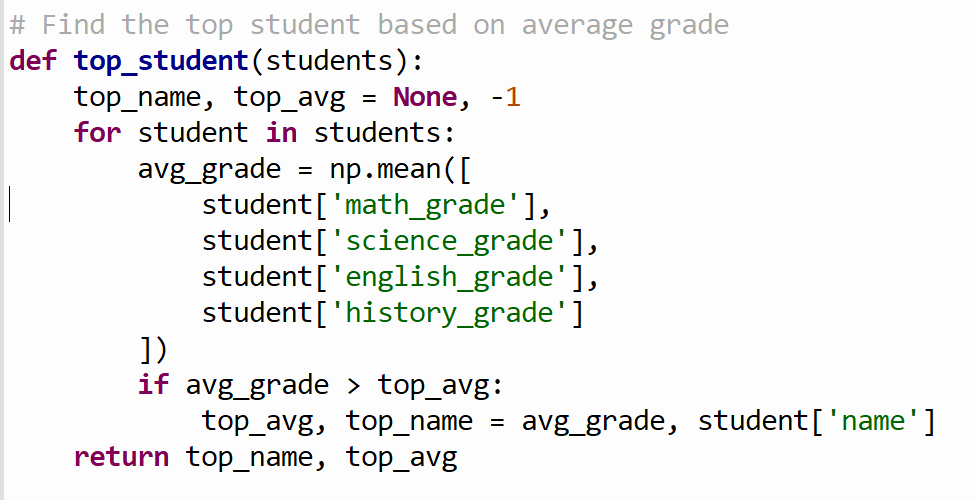
- This function finds the student with the highest average grade:
- It initializes
top_name
asNone
andtop_avg
as-1
(which ensures that any average grade will be higher initially). - For each student, it calculates their average grade.
- If the calculated average is higher than
top_avg
, it updatestop_avg
and stores the student's name intop_name
. - The function returns the name of the top student and their average grade.
- It initializes
9. Calculate Average Grade for Each Subject:

- This function calculates the average grade for each subject across all students:
- It creates a list of subjects.
- It uses a dictionary comprehension to calculate the average grade for each subject by accessing the grades of all students.
- It returns a dictionary where the keys are the subject names and the values are the average grades.
10. Identify Subjects with Below-Passing Average Grades:
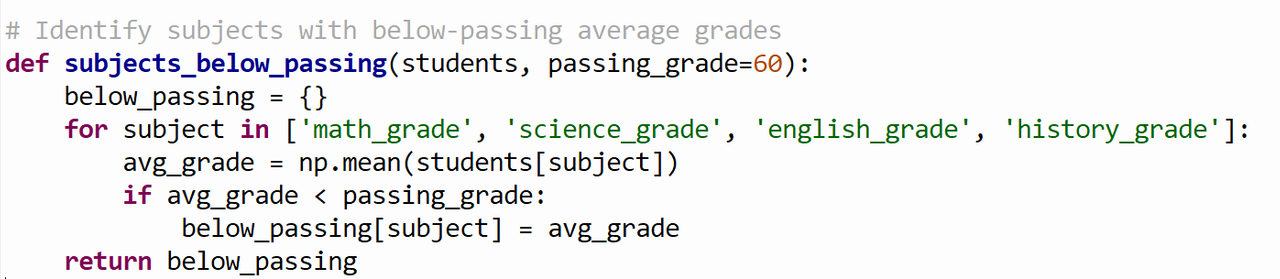
- This function identifies subjects where the average grade across all students is below the passing grade (default is 60):
- It iterates over each subject.
- For each subject, it calculates the average grade.
- If the average is below the passing grade, it is added to the
below_passing
dictionary, which is returned.
11. Display Results:
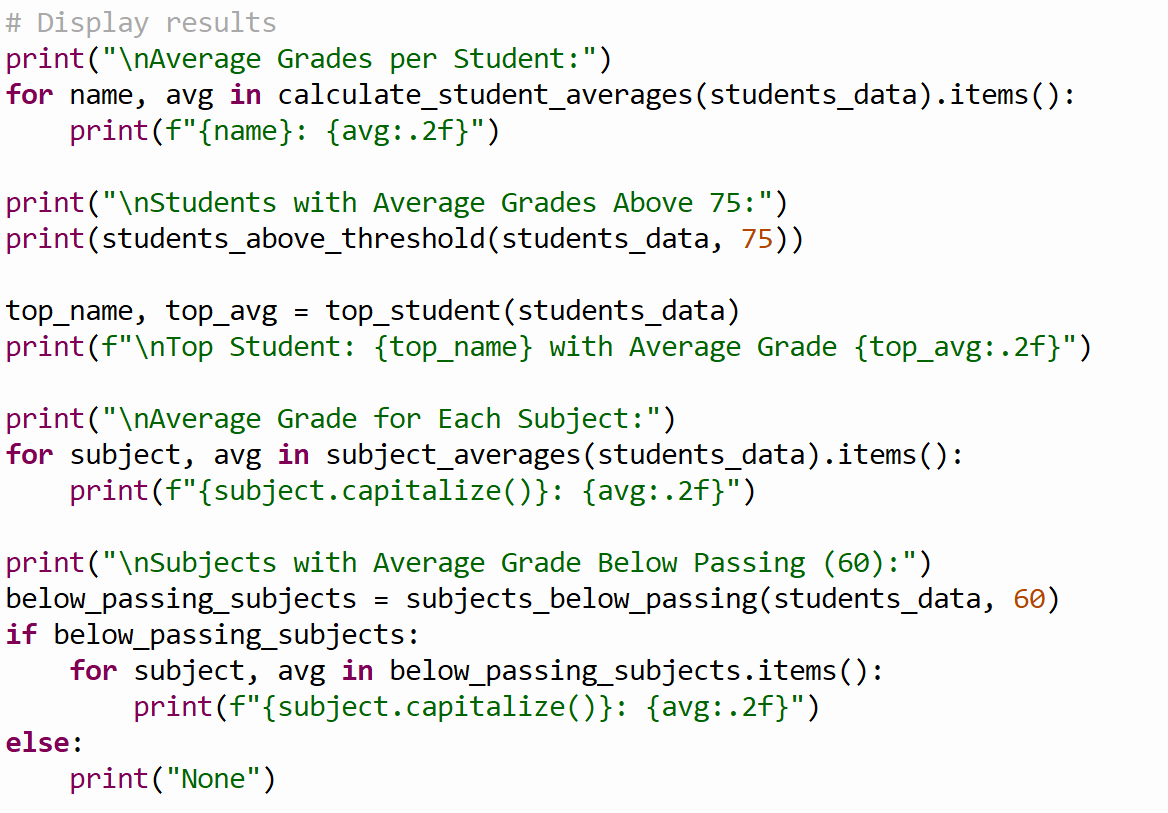
The final section of the code prints the results:
- Average Grades Per Student: Displays each student's name and their average grade.
- Students Above 75: Prints the list of students with average grades above 75.
- Top Student: Prints the name and average grade of the student with the highest average.
- Average Grade for Each Subject: Prints the average grade for each subject.
- Subjects Below Passing Grade: Prints subjects with average grades below the passing grade (60).
- The program dynamically takes input from the user for multiple students' IDs, names, and grades.
- It then processes the data to calculate averages, identify students above certain thresholds, and analyze performance by subject.
Develop an inventory management system using namedtuple to track products, including functionalities for low stock alerts and identifying high-value products.
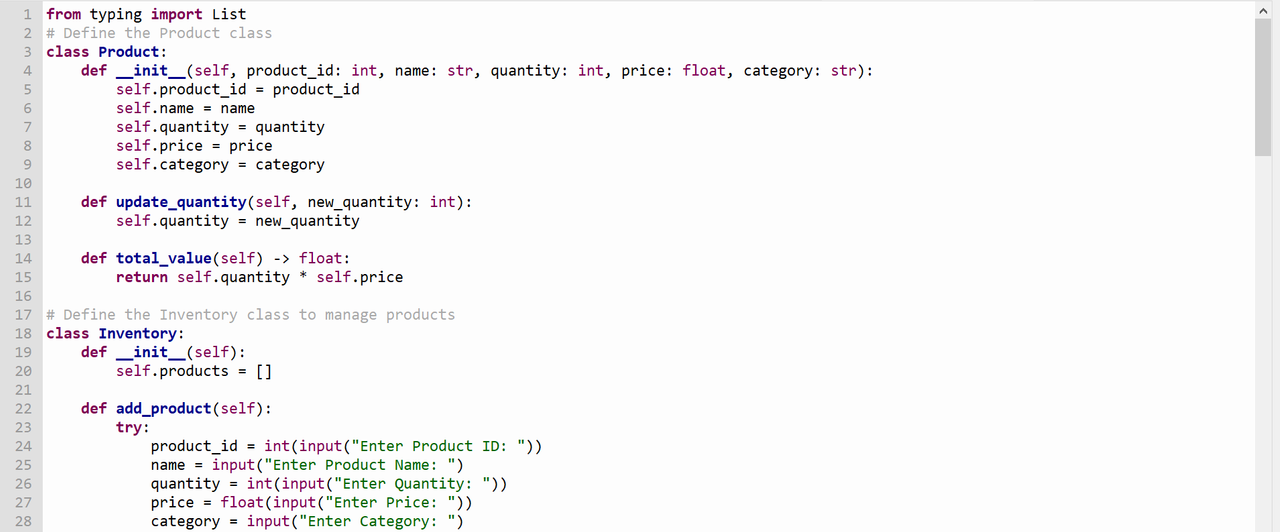
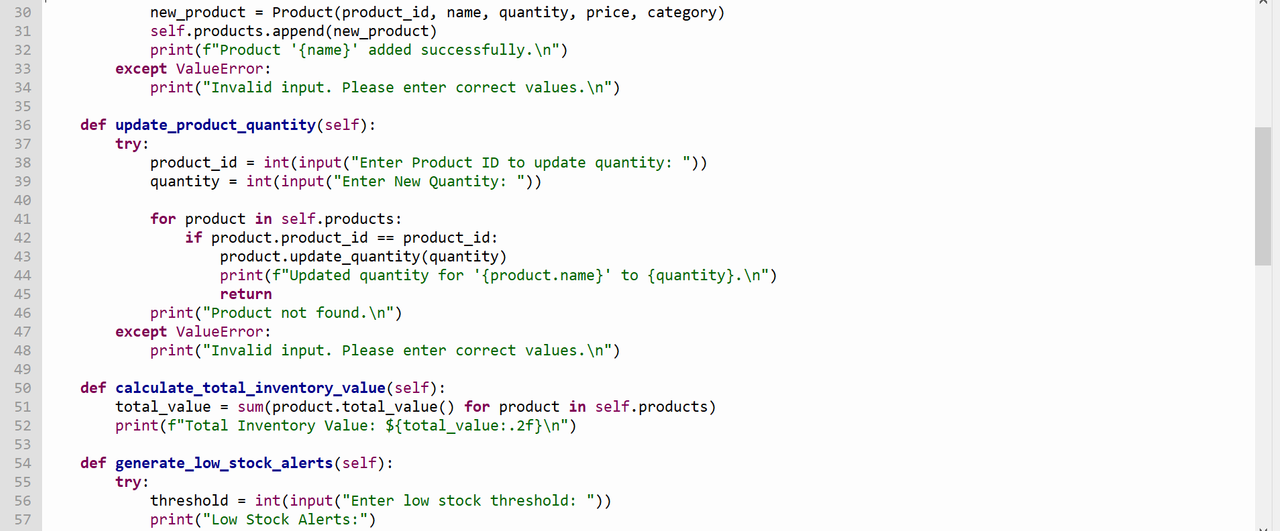
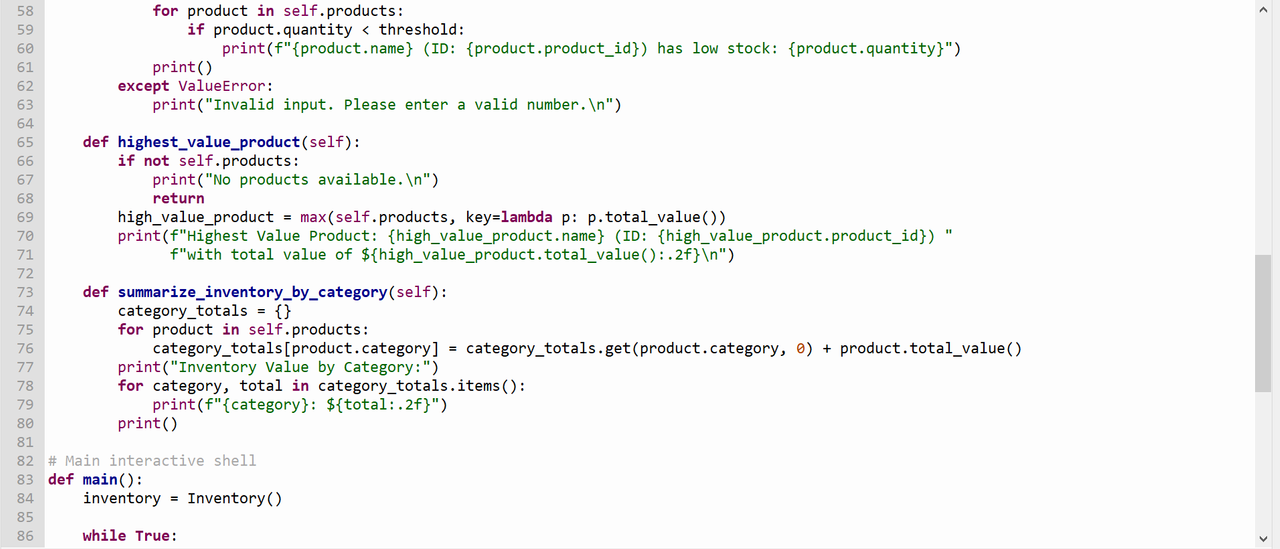
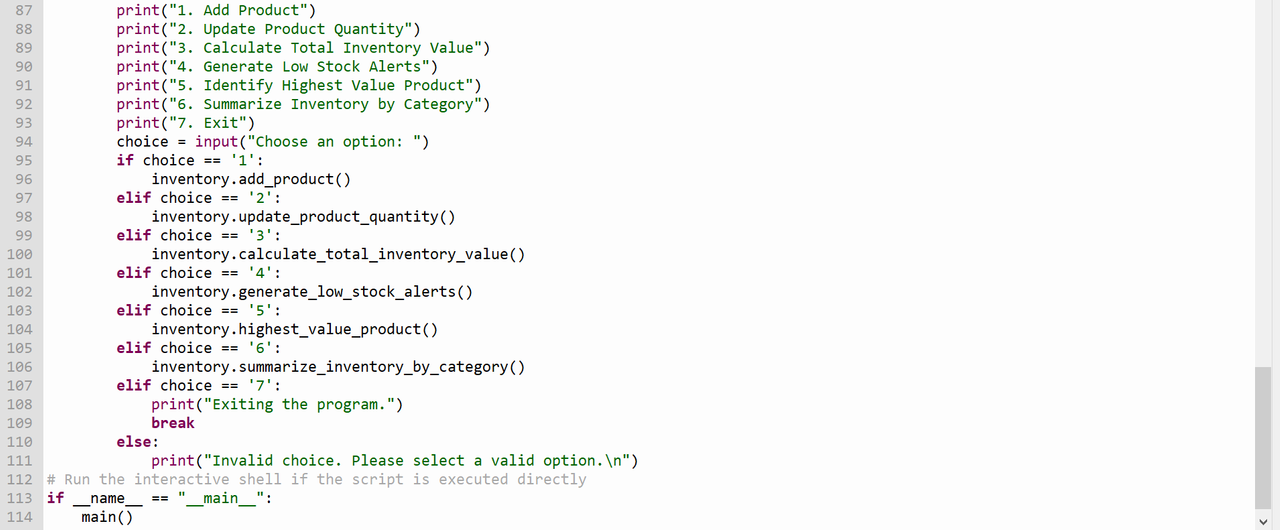
Code Breakdown
Here's a detailed explanation of the code:
1. Product Class:
The Product
class represents a product with attributes like product_id
, name
, quantity
, price
, and category
. This class also contains methods for updating product information and calculating its total value.

__init__
method: This is the constructor of theProduct
class, which is used to initialize an object of the class with attributes such asproduct_id
,name
,quantity
,price
, andcategory
.

update_quantity
method: This method allows updating the quantity of a product. It takes a new quantity as an argument and sets thequantity
attribute to the new value.

total_value
method: This method calculates the total value of the product (i.e., the product's total worth based on its quantity and price). It multipliesquantity
byprice
and returns the result as a float.
2. Inventory Class:
The Inventory
class manages the collection of Product
objects. It includes various methods to interact with the product list, such as adding a product, updating a product’s quantity, and generating reports based on inventory data.

__init__
method: This is the constructor of theInventory
class, which initializes an empty list calledproducts
to storeProduct
objects.
Method Breakdown:
add_product
:
This method allows the user to add a new product to the inventory.
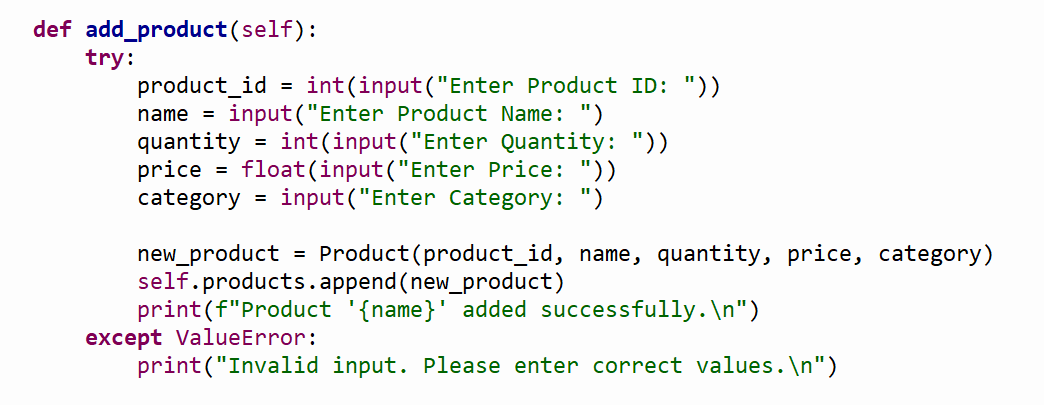
- This method prompts the user to input the details for a new product (ID, name, quantity, price, and category).
- If the inputs are valid, it creates a new
Product
object and appends it to theproducts
list. - If an invalid value is entered (e.g., a non-integer for product ID or quantity), it catches the
ValueError
and prints an error message.
update_product_quantity
:
This method allows the user to update the quantity of an existing product.
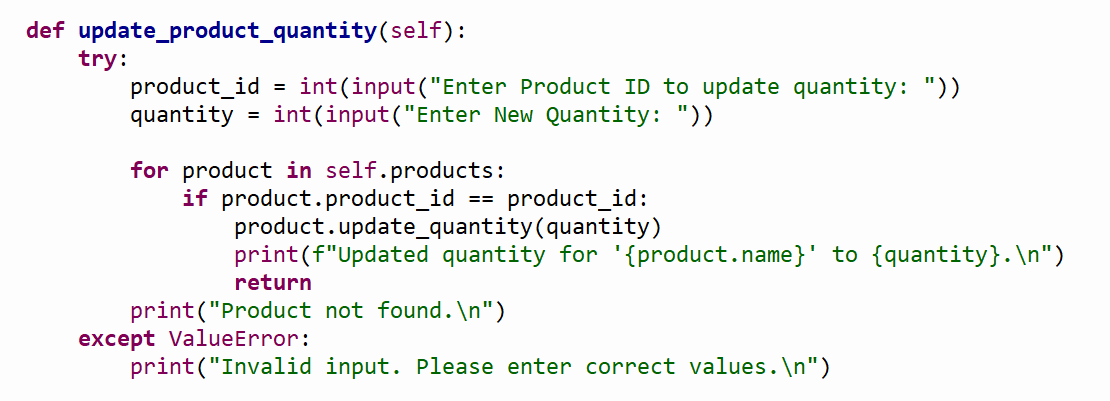
- The user inputs the product ID and new quantity.
- It iterates through the
products
list and finds the product with the matchingproduct_id
. - Once found, it updates the quantity using the
update_quantity
method of theProduct
class.
calculate_total_inventory_value
:
This method calculates the total value of all products in the inventory.

- It calculates the total inventory value by summing up the
total_value()
of each product in the list using Python'ssum()
function. - The result is printed as a floating-point number with two decimal places.
generate_low_stock_alerts
:
This method generates alerts for products with stock below a certain threshold.
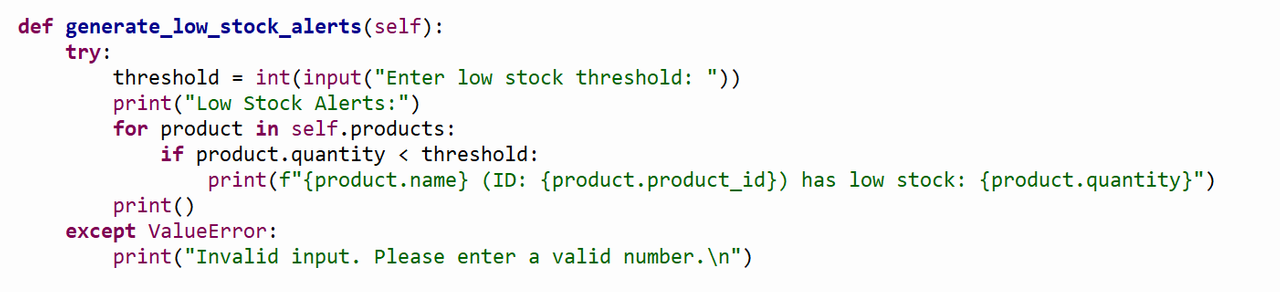
- The user inputs a stock threshold value.
- The method checks each product to see if its quantity is below the threshold. If so, it prints a low stock alert.
highest_value_product
:
This method identifies and displays the product with the highest total value (based on quantity and price).

- It uses Python’s
max()
function to find the product with the highest total value by passing a lambda function that calculates the total value of each product. - If there are no products in the list, it prints a message stating that no products are available.
summarize_inventory_by_category
:
This method summarizes the total inventory value by category.

- It iterates through all products and adds the total value of each product to a dictionary, grouped by the product category.
- Finally, it prints the total inventory value for each category.
3. Main Function:
This part contains the interactive shell that allows the user to interact with the system through a menu.
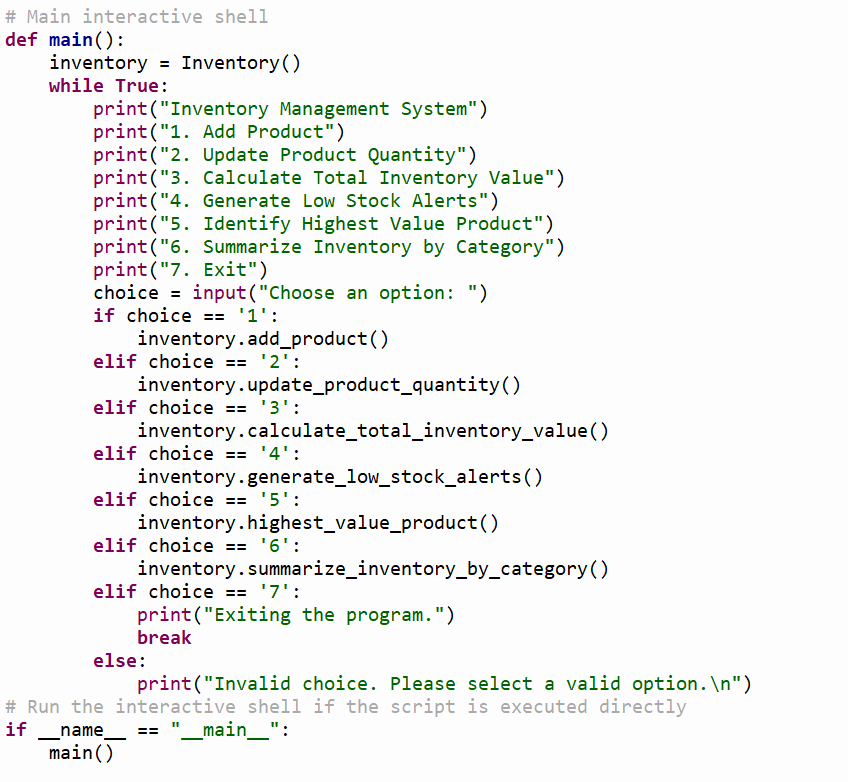
- An instance of the
Inventory
class is created to manage product data. - The menu offers the user multiple options to add products, update quantities, calculate inventory values, and more.
- Based on the user's input, the corresponding method from the
Inventory
class is called.
Create a program to process customer orders using NumPy's structured arrays, including functionalities for calculating order totals, identifying large orders, and analyzing customer purchasing patterns.
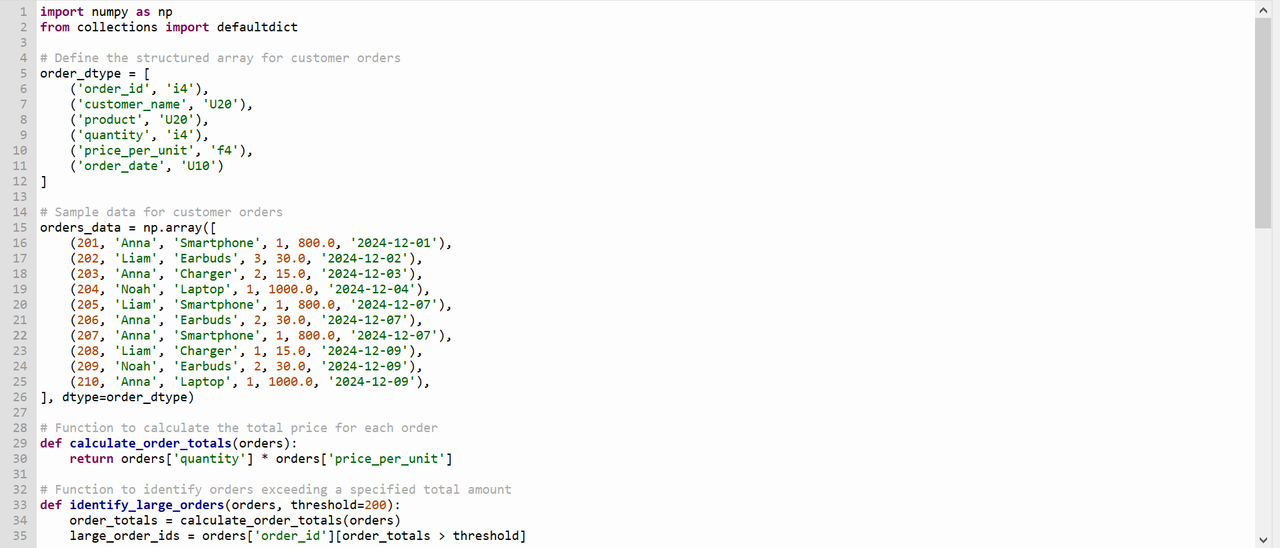
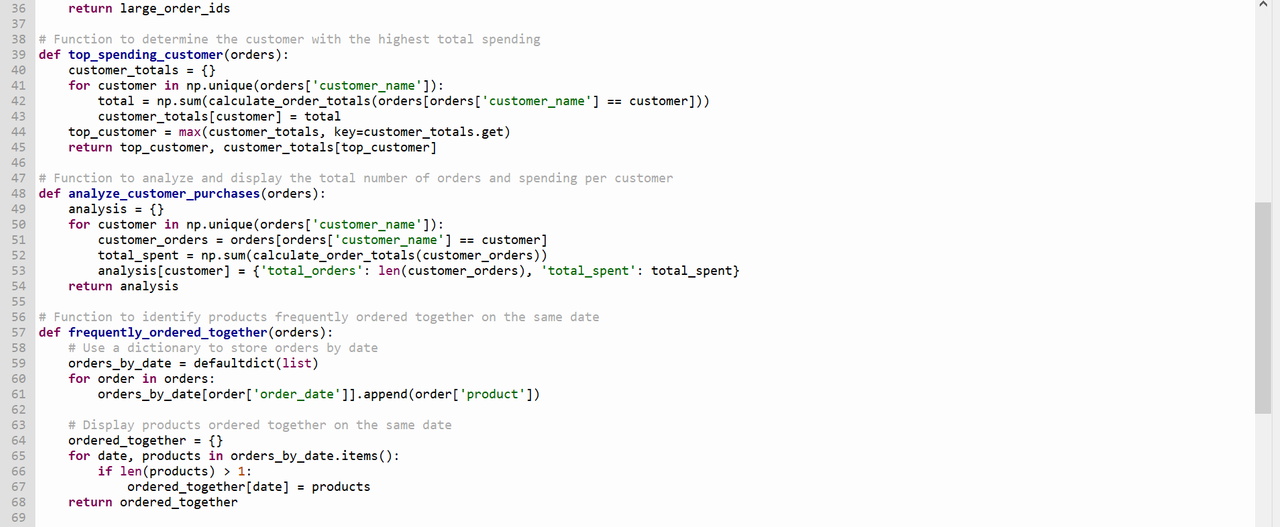
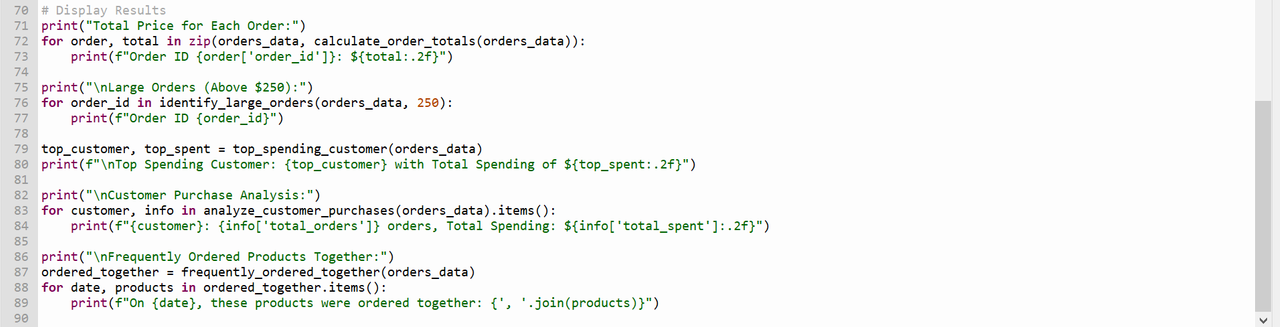
This Python code processes customer orders using NumPy’s structured arrays to handle data and functions for calculating order totals, identifying large orders, analyzing customer purchases, and finding frequently ordered products.
Code Breakdown
Here’s a breakdown of each section:
1. Imports

numpy
: Used for numerical operations and structured arrays.defaultdict
: Helps organize orders by date, allowing easy grouping of products ordered on the same date.
2. Define Structured Array
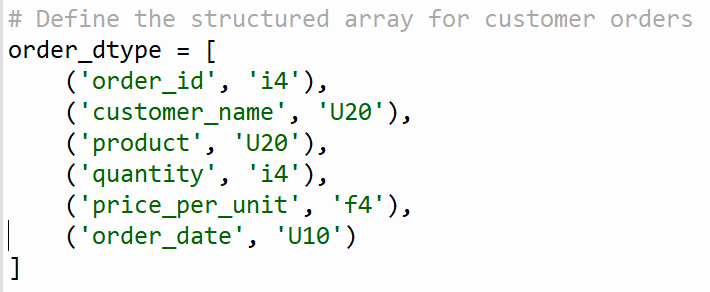
order_dtype
: Defines the structure of theorders_data
array. Fields includeorder_id
,customer_name
,product
,quantity
,price_per_unit
, andorder_date
with specified data types.
3. Sample Data
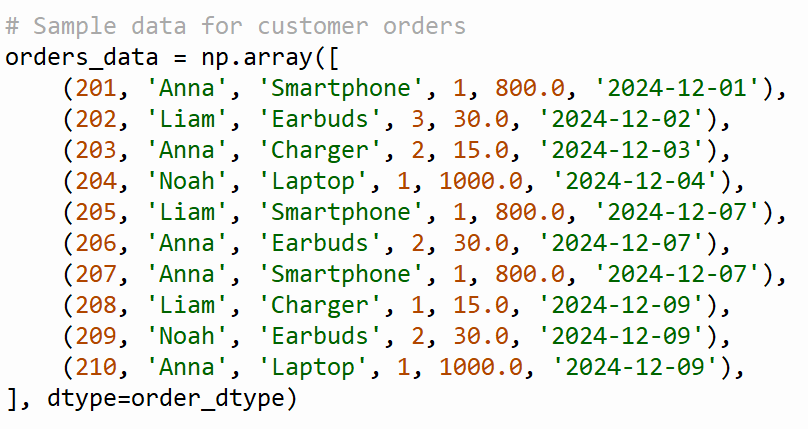
orders_data
: This array contains sample order data, each entry matching the fields inorder_dtype
. NumPy will enforce data types for each field, ensuring consistency.
4. Functions
Calculate Total Price for Each Order

- Calculates the total price by multiplying
quantity
andprice_per_unit
for each order. - Array of total prices for each order in
orders
.
Identify Large Orders

- Identifies orders where the total price exceeds a specified threshold.
threshold
(default is 200).- Array of
order_id
s where the total price is above the threshold.
Top Spending Customer
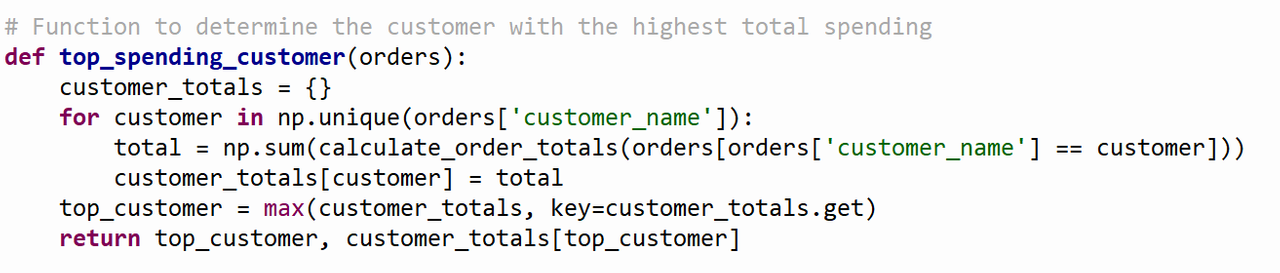
- Determines the customer who spent the most on orders.
- Program iterates through unique customers, calculates total spending for each, and identifies the highest spender.
- The name of the top-spending customer and their total spending amount.
Customer Purchase Analysis
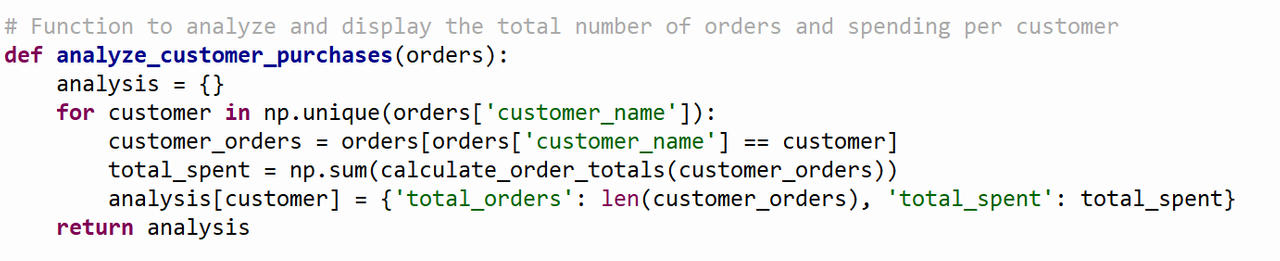
- Analyzes each customer’s total orders and spending.
- Dictionary where each customer has data on
total_orders
andtotal_spent
.
Frequently Ordered Products Together
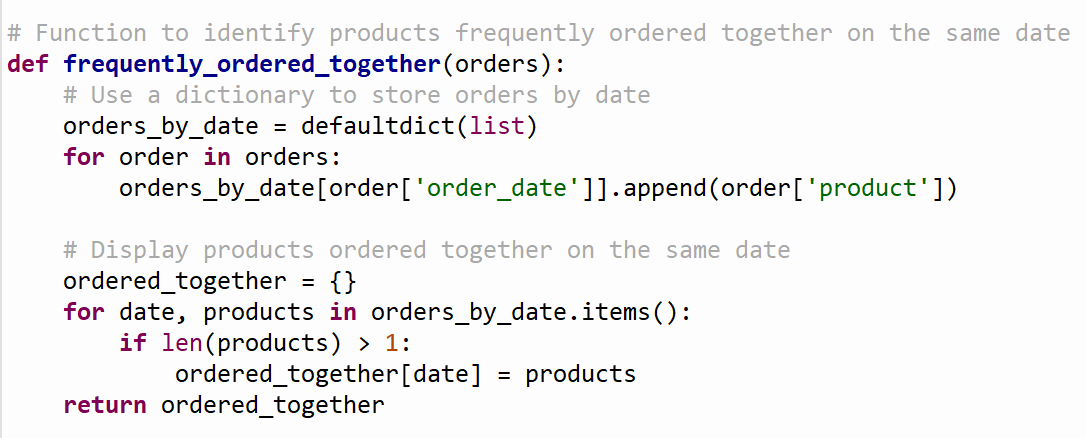
- Identifies products that were ordered on the same date, assuming orders with more than one product are frequently ordered together.
- Dictionary with dates as keys and lists of products ordered together as values.
5. Display Results
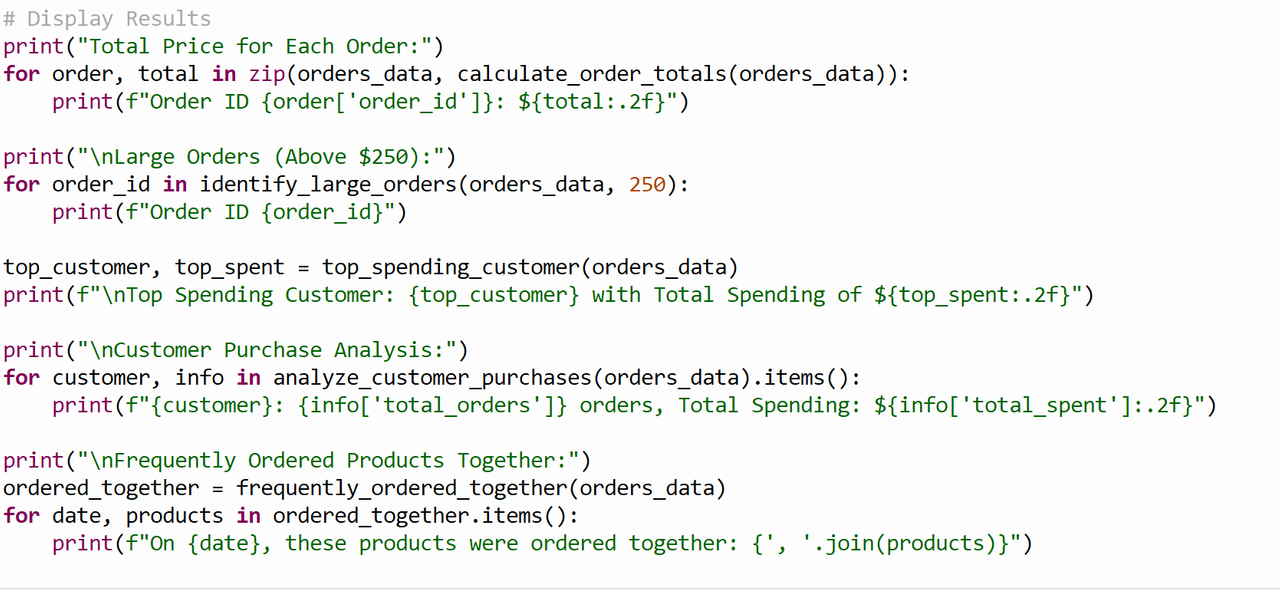
- Order Totals: Prints each order’s
order_id
and calculated total price. - Large Orders: Lists
order_id
s of orders with totals above a threshold (250 in this case). - Top Spending Customer: Displays the customer with the highest spending and the amount spent.
- Customer Purchase Analysis: Shows total orders and spending per customer.
- Frequently Ordered Products Together: Lists dates and products ordered together on those dates.
This structured approach allows flexible processing of the customers orders.
Analyze weather data using dataclass and NumPy, including functionalities for calculating averages, identifying trends, and detecting anomalies
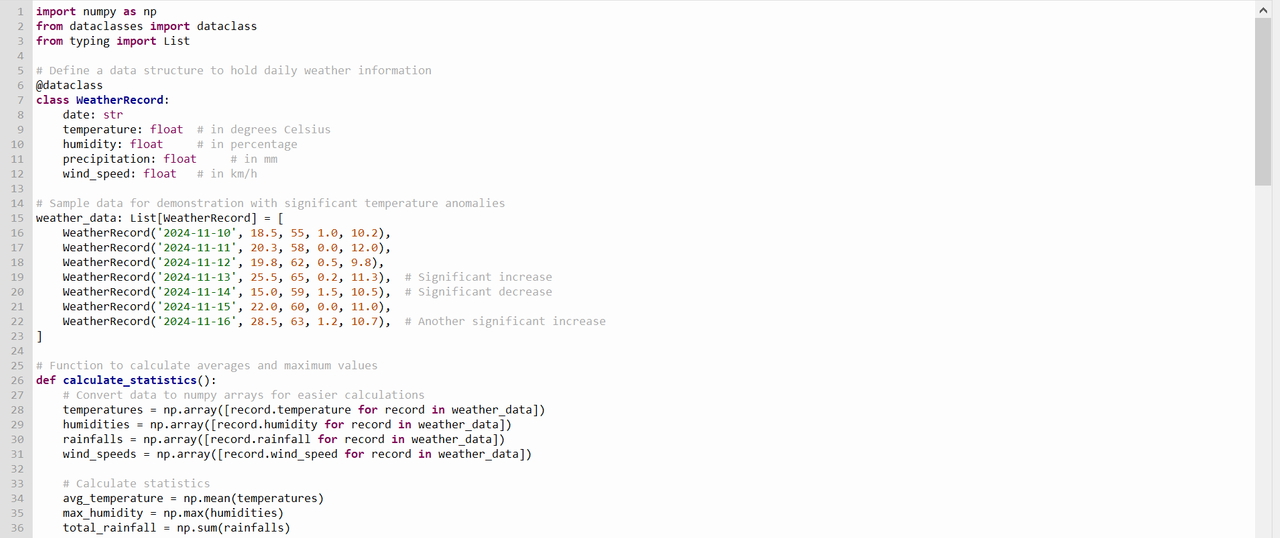
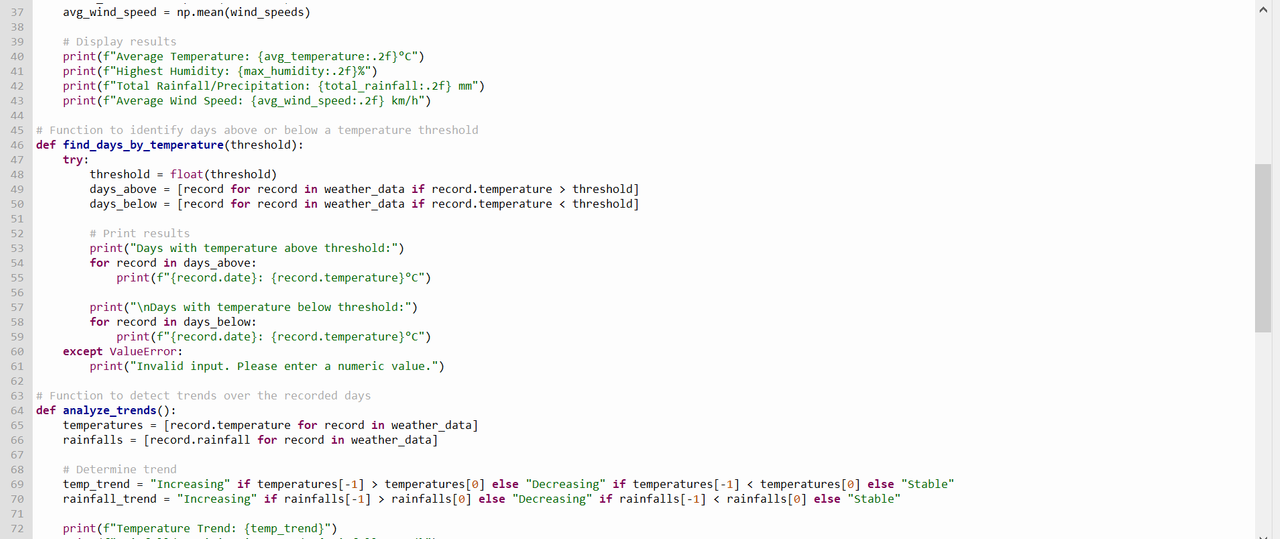
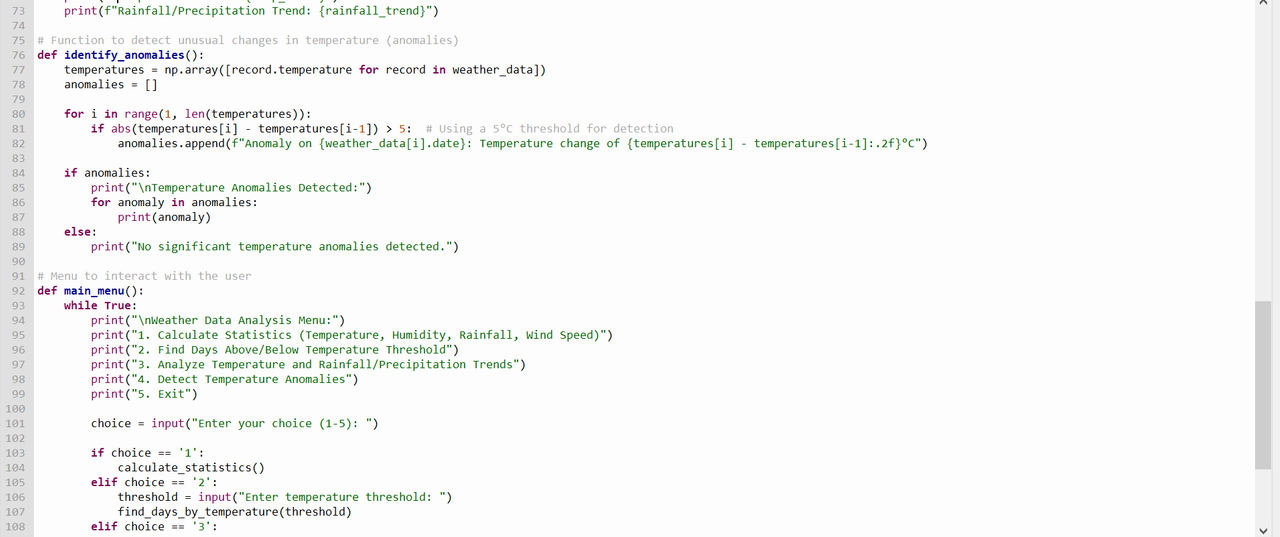

This code analyzes daily weather data, performing various operations like calculating statistics, finding temperature threshold days, analyzing trends, and detecting temperature anomalies.
I have divided the complete code into 8 major parts. Here is a breakdown of its components:
1. Importing Libraries
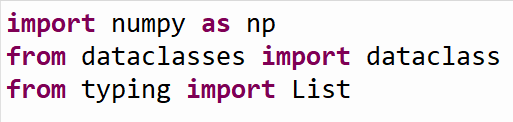
- NumPy (
np
): A library used here for efficient array operations. - dataclass: A decorator from Python’s
dataclasses
module, used to define theWeatherRecord
class in a concise way. - List: A typing hint for lists, ensuring the data type of
weather_data
.
2. Defining the Weather Data Structure
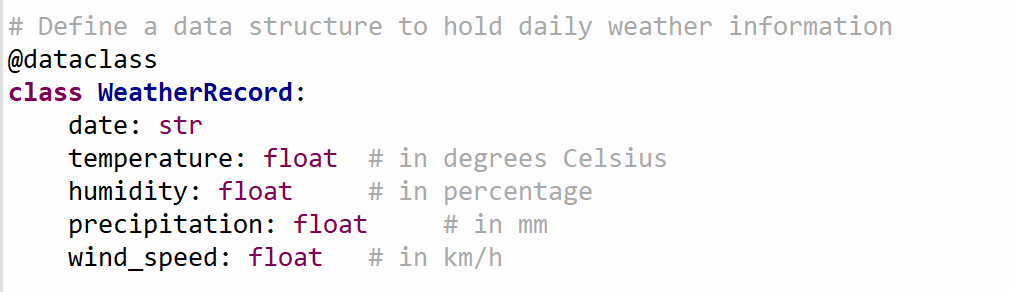
The WeatherRecord
class represents a day’s weather data with fields for date
, temperature
, humidity
, precipitation
, and wind_speed
.
3. Sample Data
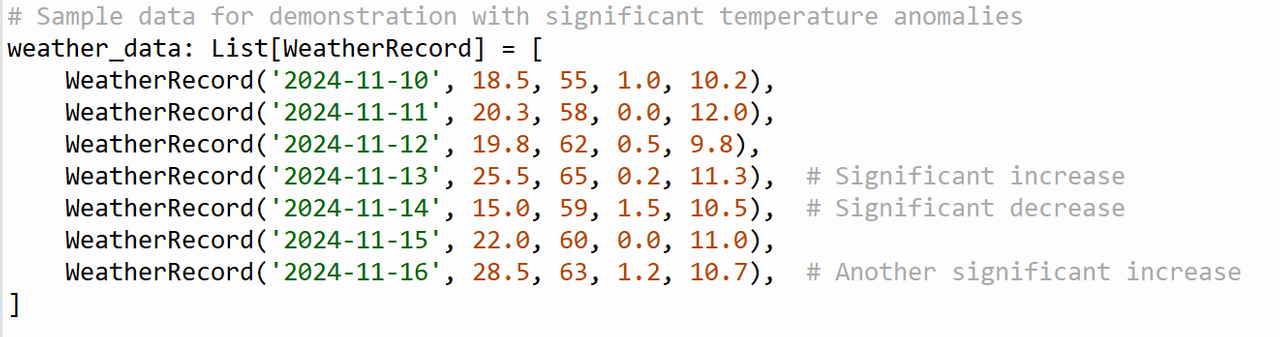
This list of WeatherRecord
objects holds sample weather data. Specific days have significant temperature changes, which will trigger the anomaly detection.
4. Calculating Statistics
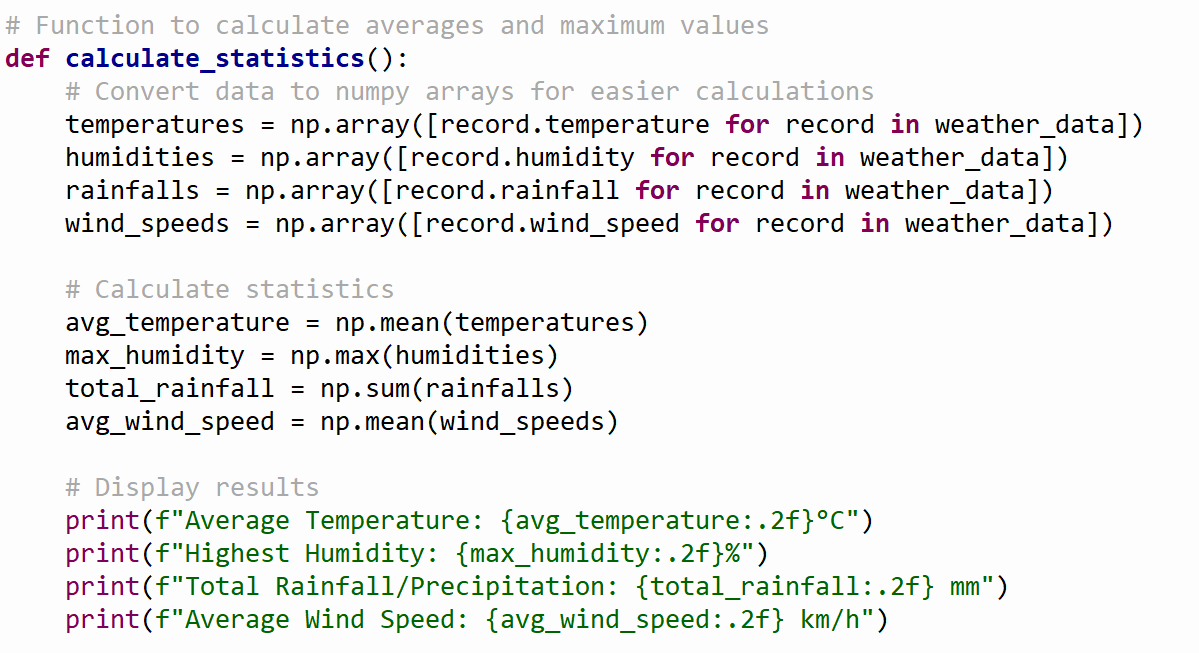
- Calculates and prints average temperature, highest humidity, total precipitation, and average wind speed.
- Extracts each weather attribute into separate arrays.
- Uses NumPy functions like
mean
andmax
for calculations.
5. Finding Days Above/Below a Temperature Threshold
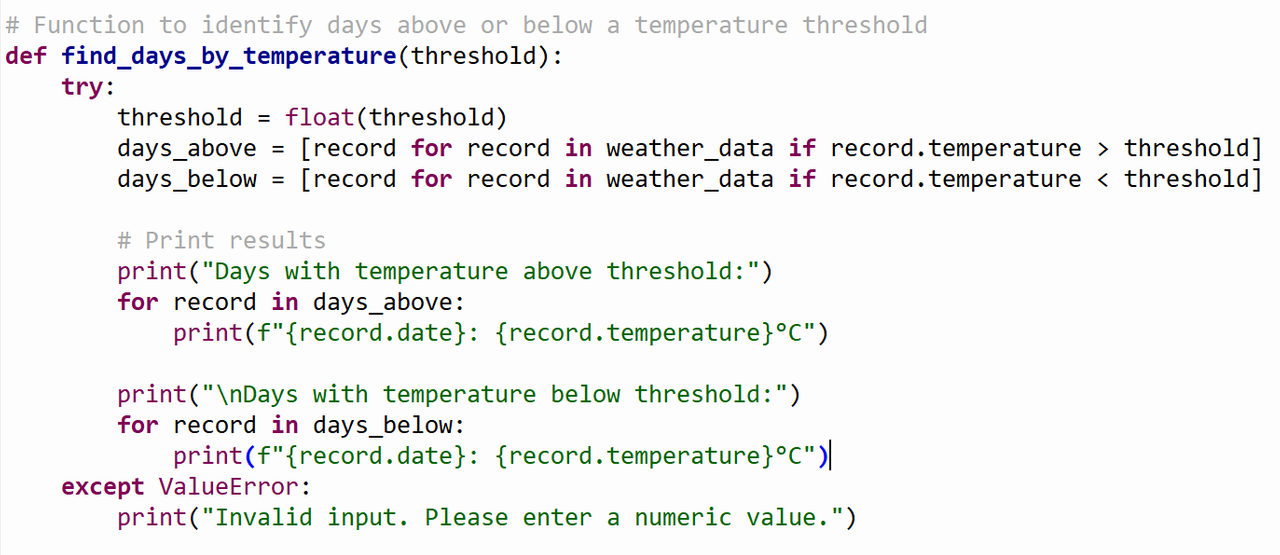
- Lists days with temperatures above or below a user-specified threshold.
- Filters
weather_data
based on the threshold. - Prints results in two categories: above and below the threshold.
6. Analyzing Trends

- Determines whether temperature and rainfall show an increasing, decreasing, or stable trend over time.
- Compares the first and last values of
temperatures
andrainfalls
. - Prints the trend for each metric.
- Compares the first and last values of
7. Detecting Temperature Anomalies
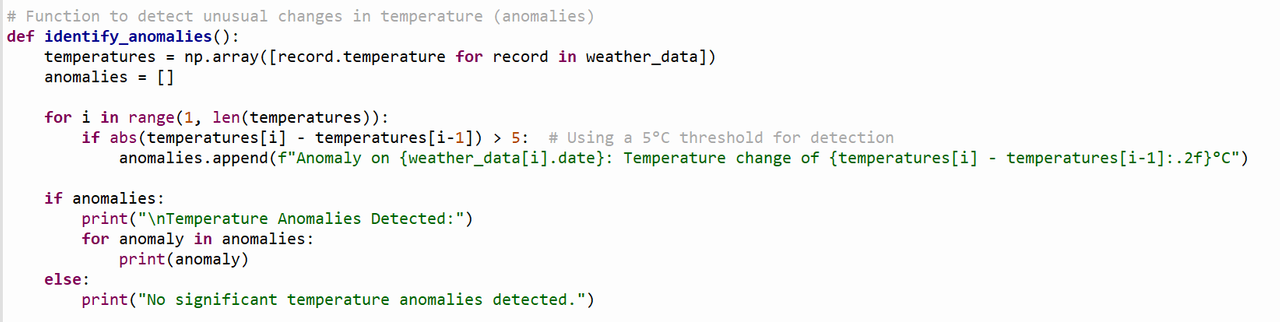
- Identifies days where temperature changes by more than 5°C compared to the previous day.
- Loops through
temperatures
, checking if the absolute difference with the previous day exceeds 5°C. - Stores anomalies in a list and prints them if found.
8. Main Menu for User Interaction
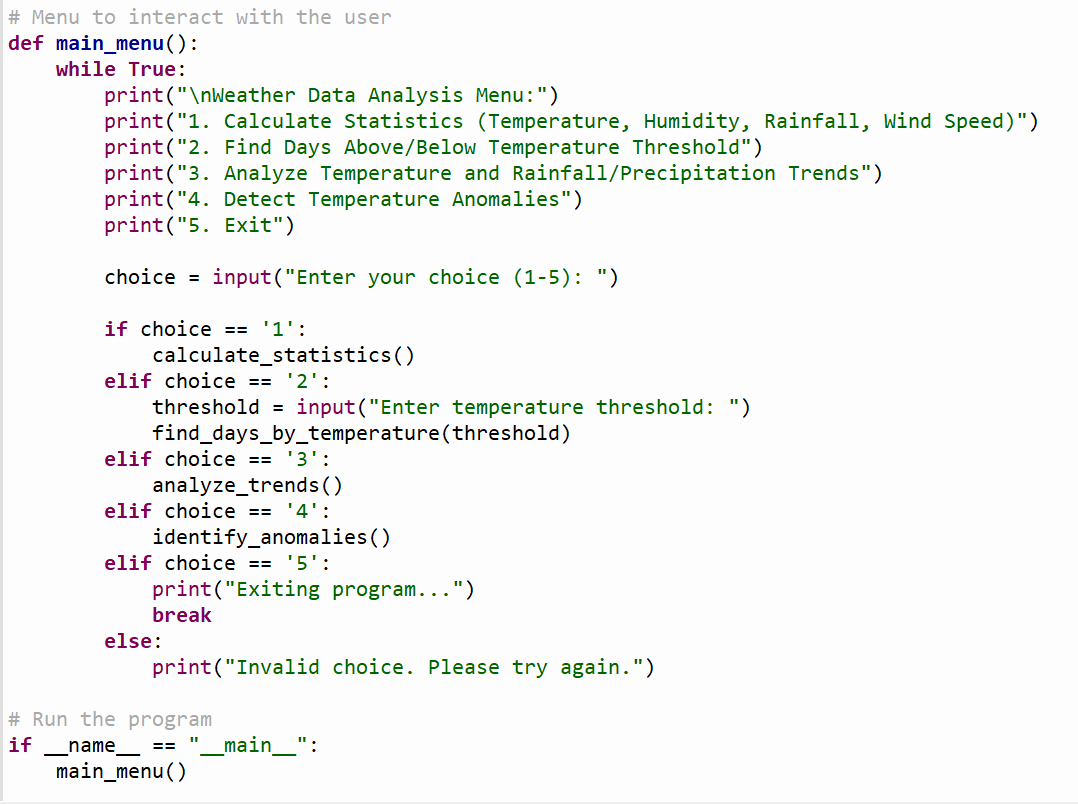
- Provides an interactive menu for users to choose different analysis options.
- Calls appropriate functions based on user input.
I invite @wirngo, @josepha, @memamun to join this learning challenge.
Comments