SLC S22 Week1 || Getting Started with Java and Eclipse
4 comments
The first Homework of the @kouba01's Java class, if you want to give it a try you can find it here.
Let's get started.
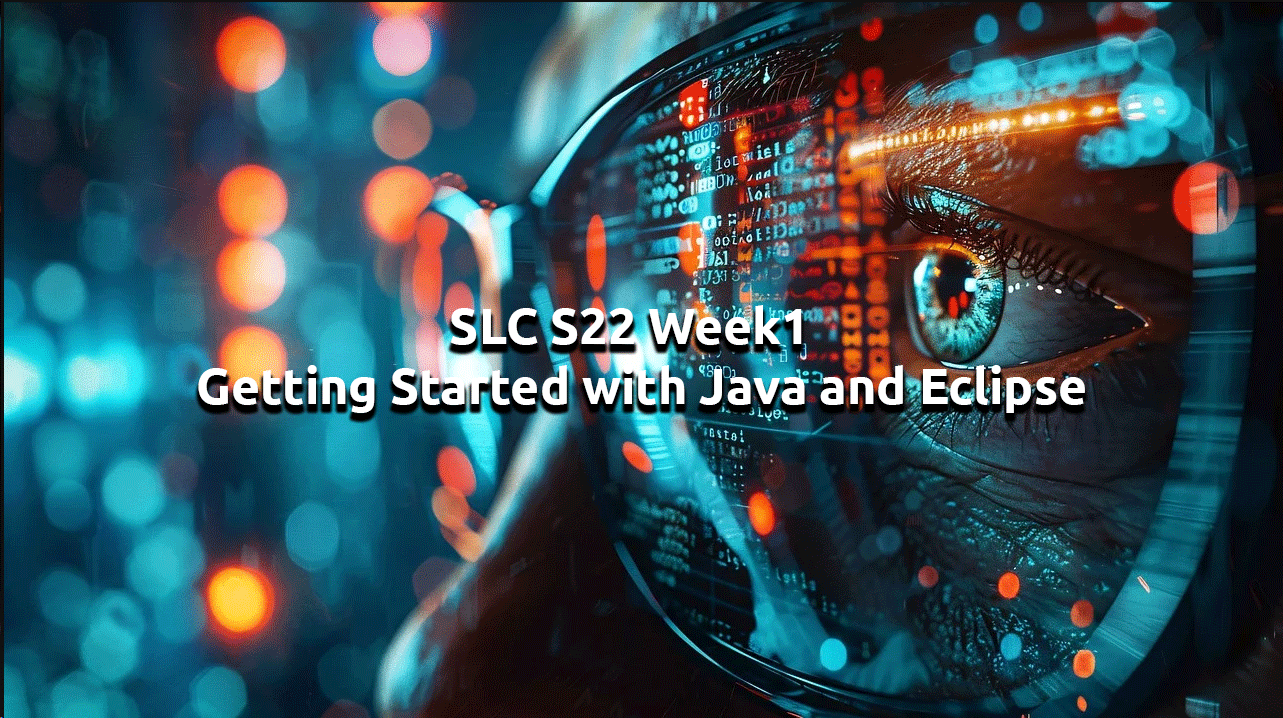
Image taken from Pixabay
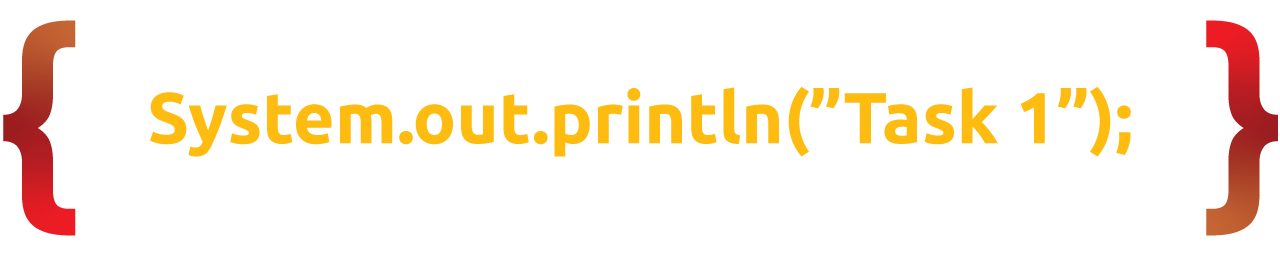
Write a detailed explanation of the differences between JDK, JRE, and JVM by including their roles, functionalities, and interdependence. Add graphic illustrations which cover this points :
- JDK: Development tools, includes JRE and compiler.
- JRE: Execution environment for Java programs.
- JVM: Virtual Machine responsible for running Java bytecode.
Java programming is composed of 3 core technology packages, JDK [Java Development Kit], JVM [Java Virtual Machine] and JRE [Java Runtime Environment].
Let's take them one by one and see what each does:
Acronym | Description |
---|---|
JDK | Also known as Java Development Kit is a kit/bundle that provides a platform/environment for building Java applications, applets and components it includes a collection of tools and libraries needed to create and run Java based software. Used by developers for running, debugging, writing and compiling Java programs. It basically generates bytecode from Java source files and runs them. |
Acronym | Description |
---|---|
JRE | Also known as Java Runtime Environment is a package that contains the runtime environment that executes Java programs, a key part of Java platform, used to run applications written in Java. Runs compiled Java using the JVM. |
Acronym | Description |
---|---|
JVM | Also known as Java Virtual Machine, is responsible for running the bytecode that is produced after compilation. It is required by any machine that runs/will run Java programs, it comes included in the JDK and JRE installation. It's main components are, a bytecode interpreter, JIT compiler (Just-in-time) and a garbage collector. |
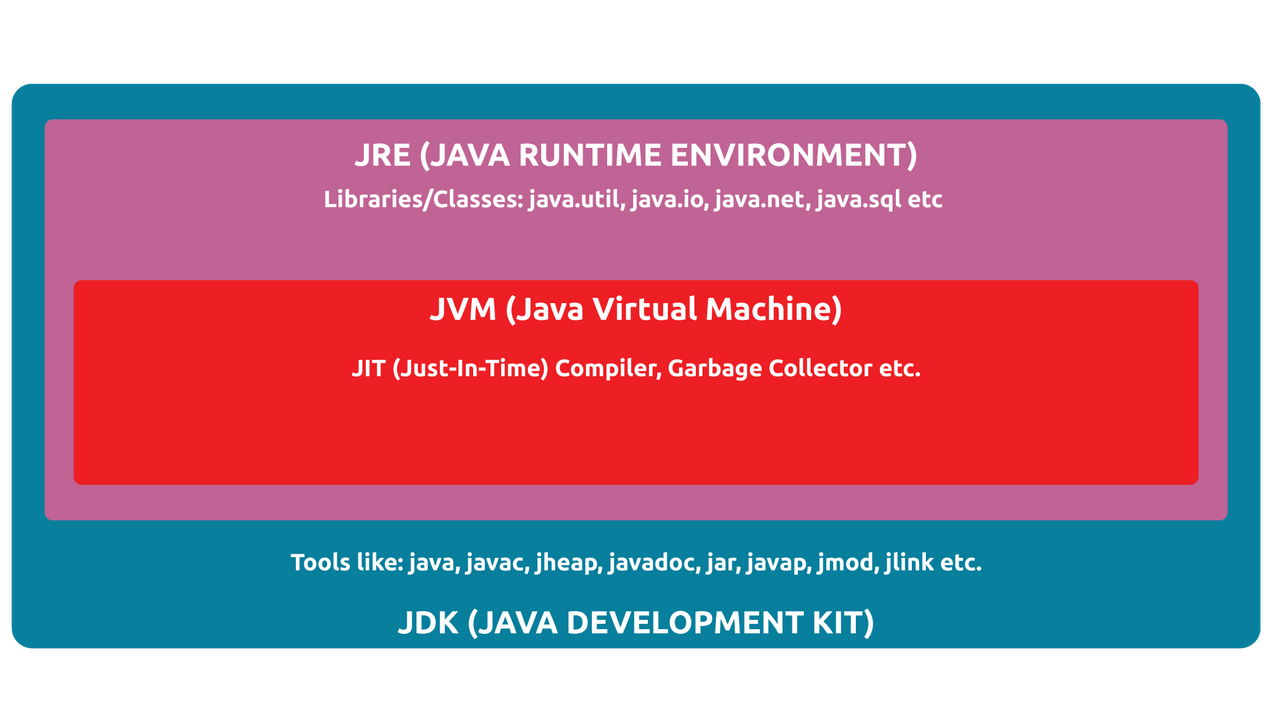
Illustration of JDK, JRE, JVM
The process is as it follows:
You write your code, when you compile you invoke the javac
compiler from JDK, this converts your code to bytecode
and the .java
file is converted to a .class
one. Here the compiler checks for syntax errors only. When you compile it JRE provides the needed libraries and/or packages and calls the JVM to run the program. JVM prepares the .class file to be executed, it loads the file in the memory and the the bytecode is being verified, if everything is ok the code gets executed.
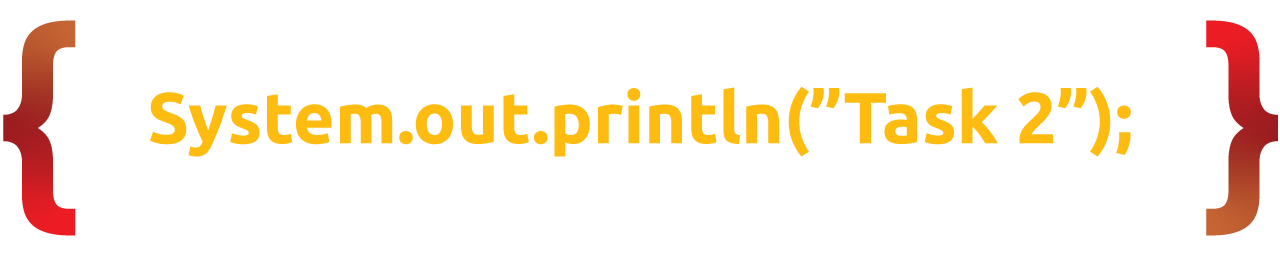
Install and Set Up Your Java Development Environment and provide step-by-step screenshots of the installation process, Eclipse configuration.
The install process wasn't that hard, I've worked previously with the JDK, it is also required by Unity if you work on mobile games.
Let's see the installation process step-by-step.
Went to the Oracle's official website provided by @kouba01, selected Windows as this is the platform I am using and downloaded the installer.
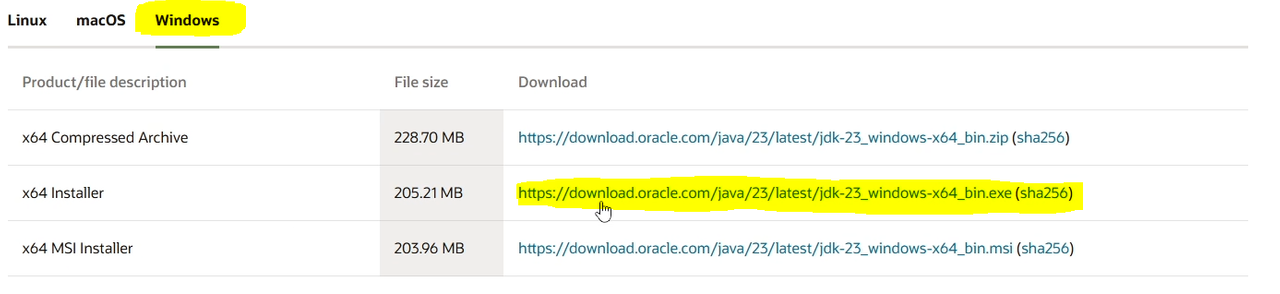
Once the download was ready, I ran the installer and followed the installation steps provided by the setup (steps in the GIF below).
I kept the default Path, changing these usually ends bad, had issues with it in Unity where errors occurred because Unity was searching for components in these default paths.
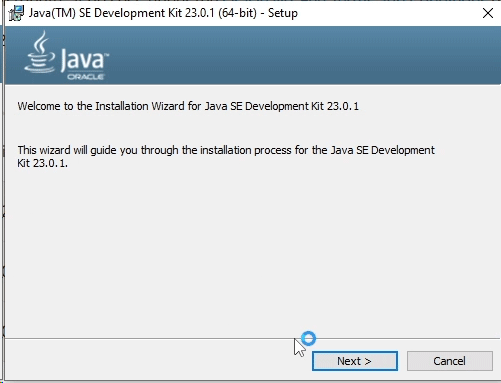
JDK was now ready, time to install Eclipse. Again went to Eclipse official website via @kouba01's link and downloaded the installer.
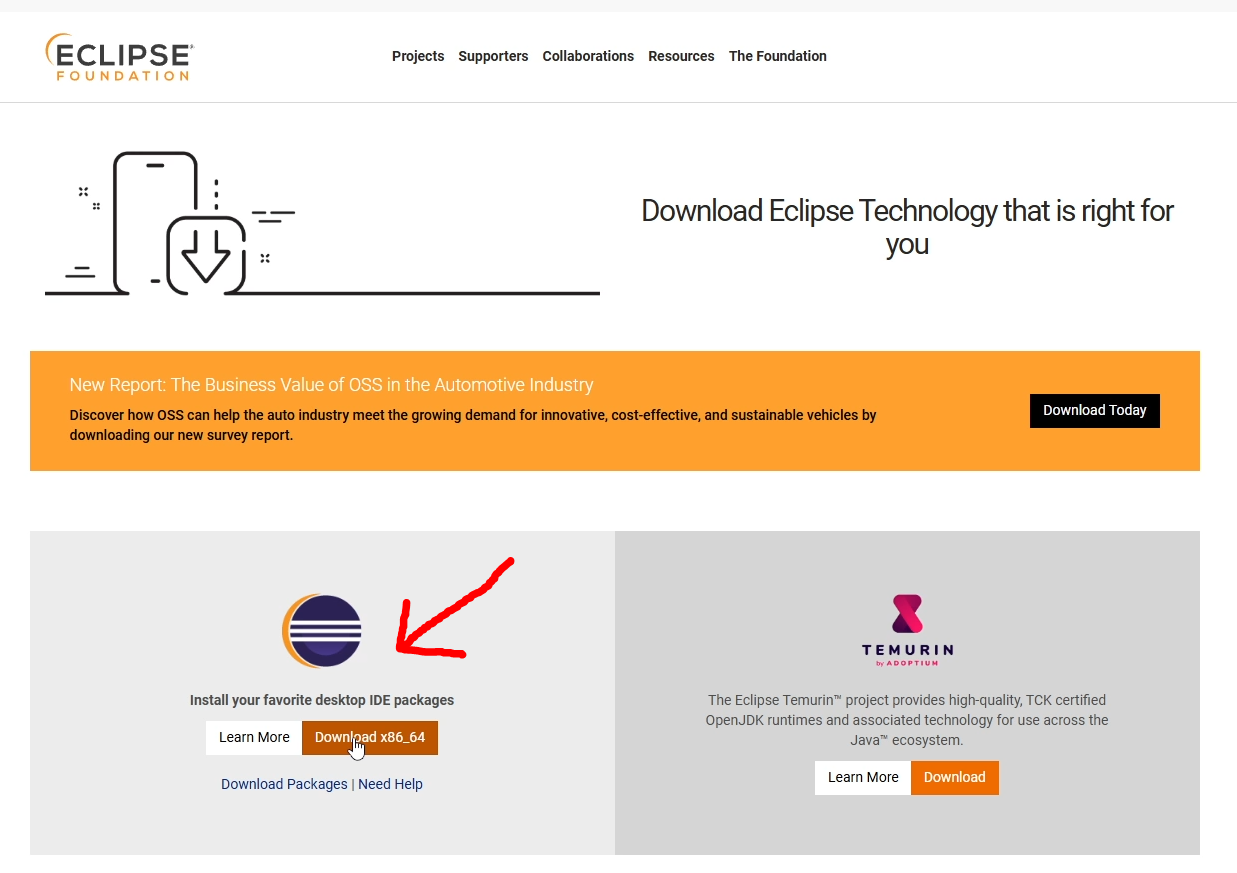
Once the installer was fully downloaded and ready, I ran it and followed the installation process, selected Eclipse IDE of Java Developers
, left the default paths for the installer, accepted the user agreement and the installation process started.
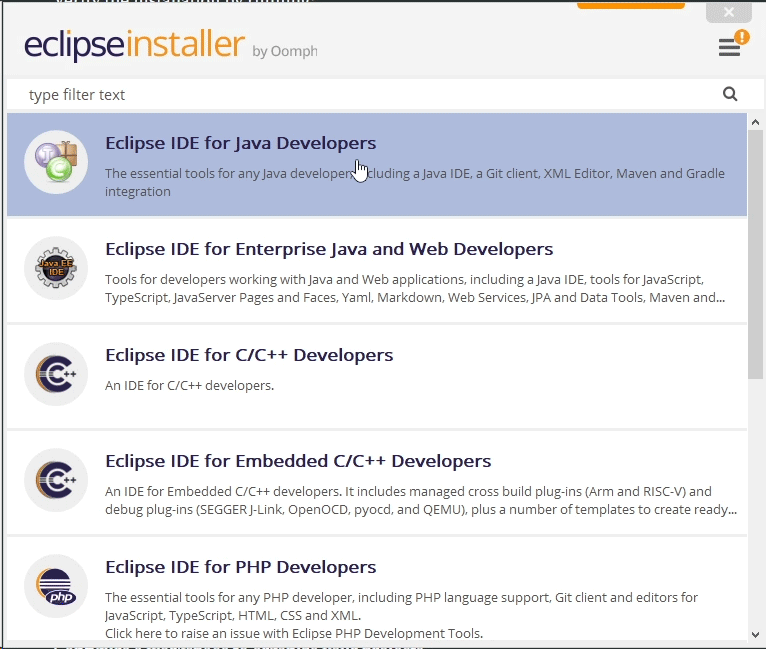
Everything was ready, it was time to see if everything was installed correctly and we can run Java code.
With Eclipse freshly opened, I went to create a new project.
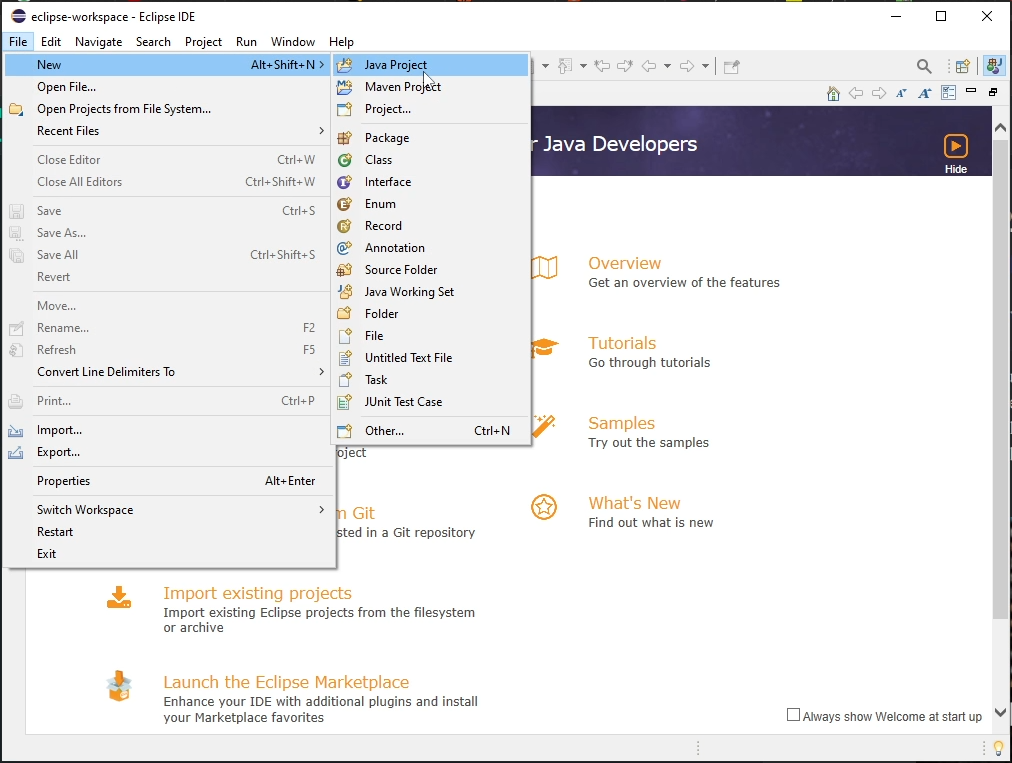
Named it test
.
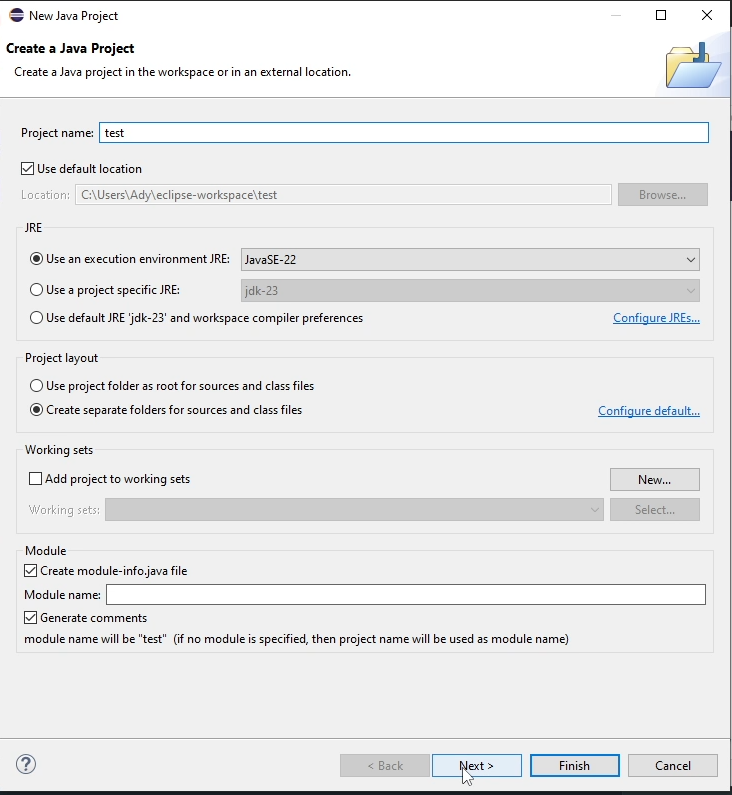
With the project created it was all that was still needed was a class for the code, so I went and created one, MyFirstProgram
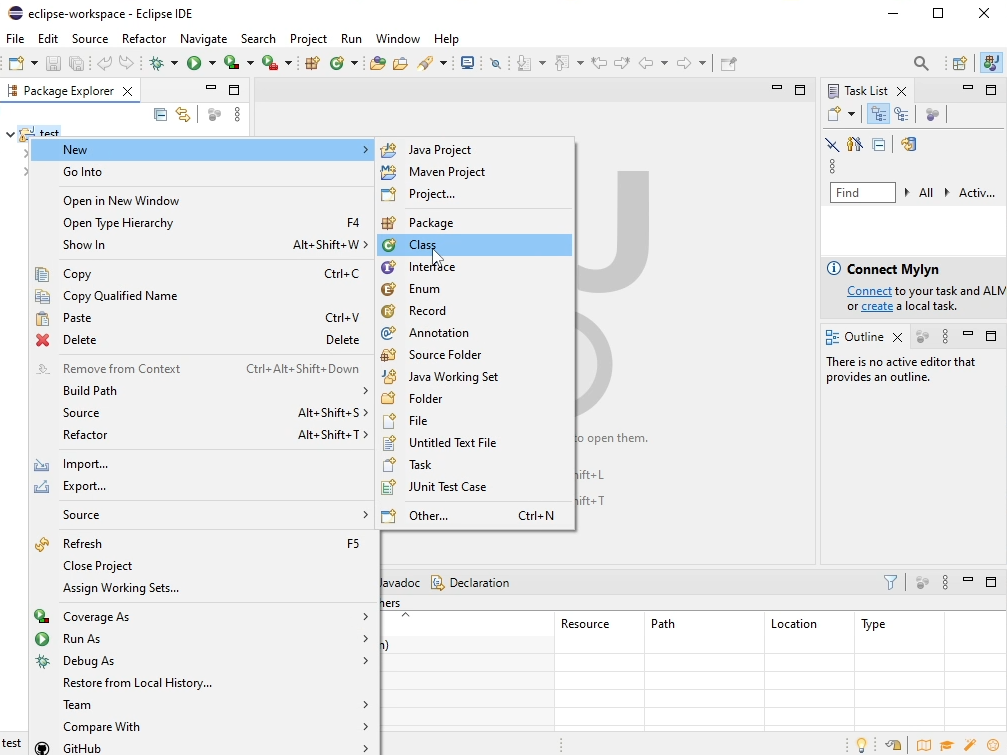
In the generated class I added a System.out.println("Hello Steemit!!!");
and ran it to see if it will be compiled successfully and the string is being showed in the console. And it worked!
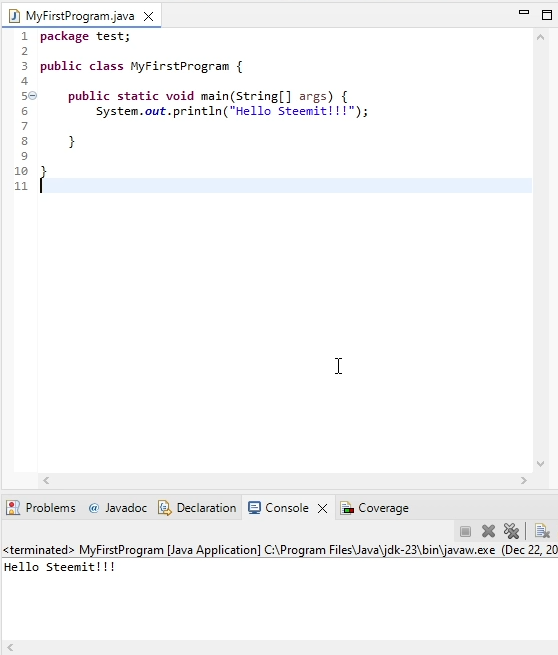
Time to move to the next tasks!
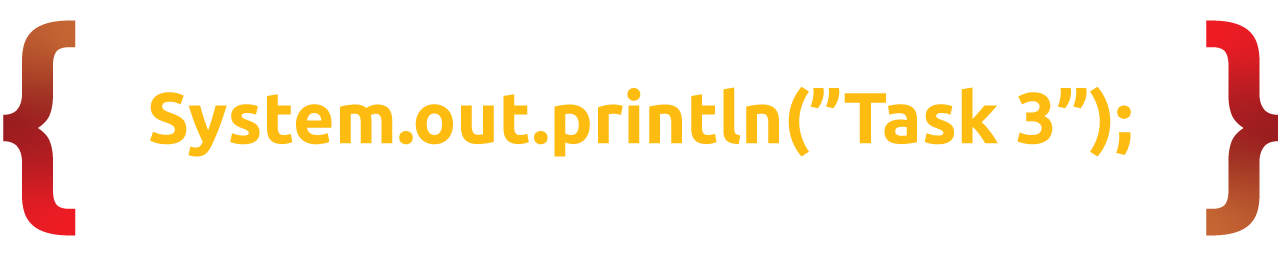
Write a program that calculates and displays the sum of the first 100 integers. Define the main method in a class named Sum to handle the entire calculation.
- 1.Save this program in a file named Sum.java, compile it, and execute it.
- 2.Create a new program named Sum2.java, where the calculation of the sum of the first 100 integers is performed in a separate function called calculateSum. This function should then be called from the main method.
I will present the code execution in GIFs down below.
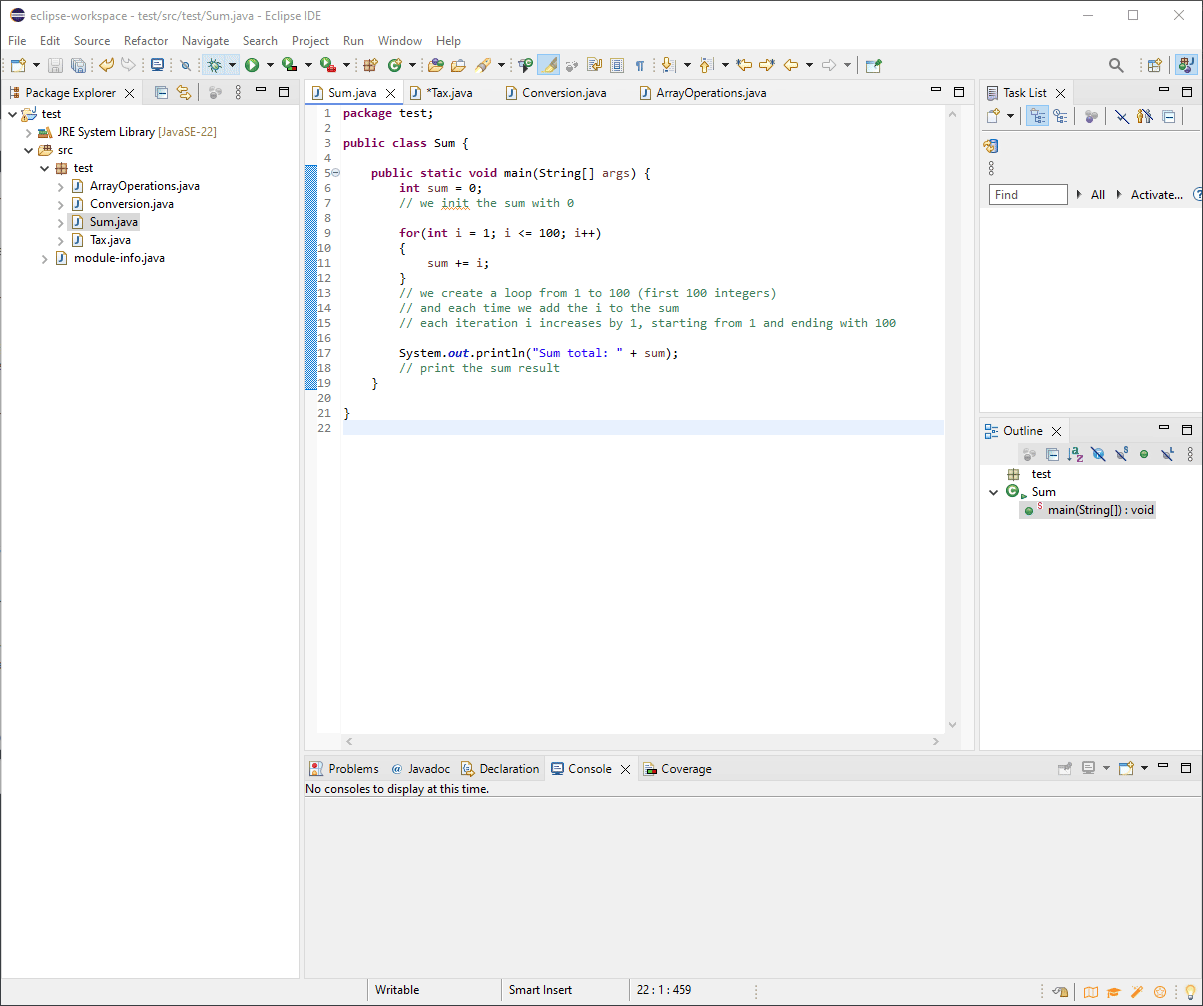
For the first Sum, I created a for loop with i
starting from to 100, each time we go thorugh the loop we add i
to the sum
. The variable sum
was initialized with 0 before the loop. Once the loop is finished we show the sum
value.
Let's move to the second sum.
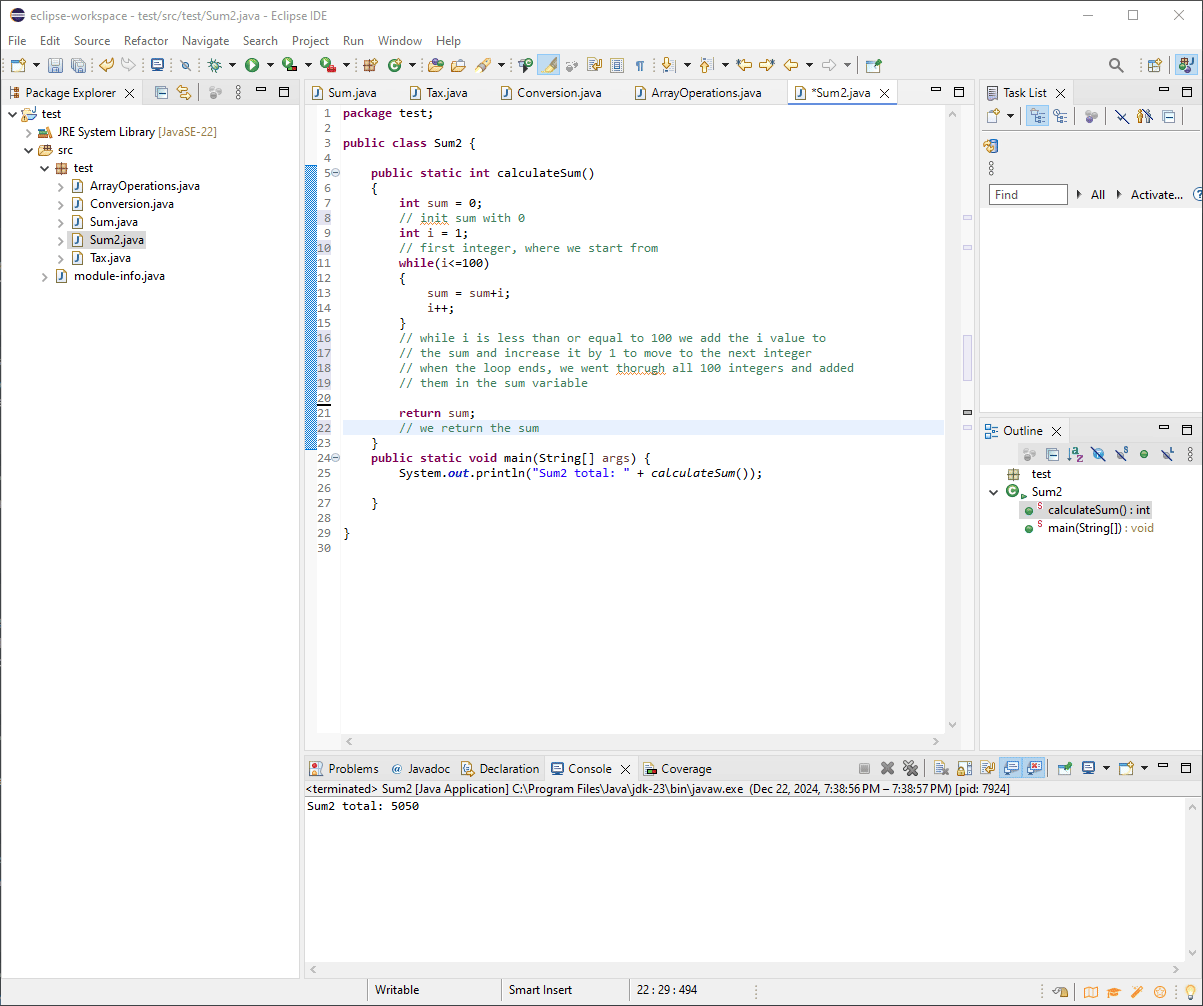
This time I changed the loop, from a for
to a while
, initialized sum
with 0 and i
with 1, and the while
starts from 1 and adds the value to sum
as long as i
is less or equal to 100.
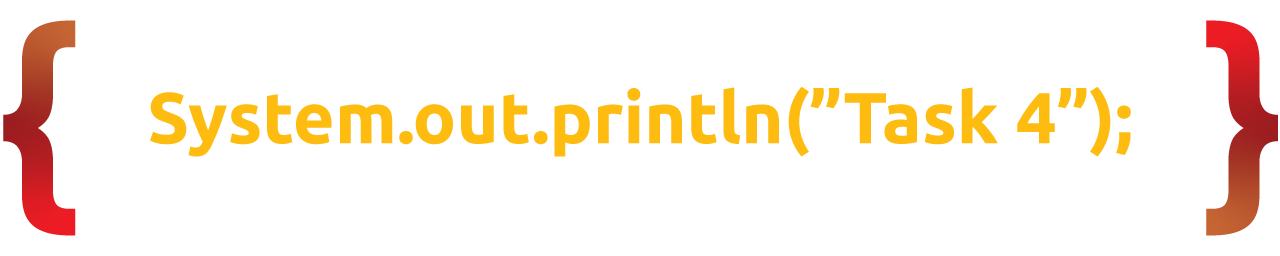
Translate, Complete, and Debug the Following Program Named Tax.java:
**
public class Tax {
public static double calculateTax(double income) {
// result = value of the tax calculated based on the income
}
public static void main(String[] args) {
System.out.print("Tax: ");
System.out.println(calculateTax(57000));
}
}
To calculate the tax, the income is divided into brackets, with each bracket defined and taxed according to the following table:
Income Bracket | T1 ≤ 20000 | 20000 < T2 ≤ 40000 | 40000 < T3 ≤ 60000 | 60000 < T4 |
---|---|---|---|---|
Tax Rate | 5% | 10% | 15% | 30% |
For an income of 57,000, the tax calculation would be as follows:
T1: 20000 * 0.05 = 1000
T2: (40000 – 20000) * 0.10 = 2000
T3: (57000 – 40000) * 0.15 = 2550
Total Tax: 5550
For this task we have 4 tiers of taxing, each one will be represented in a different if-statement. Only for the T1 we can calculate the Tax directly, for all the others we need to add the previous Tax Tier and substract 20k-40k-60k from the income to get the real value that needs to be multiplied to get the tax.
Also I've added a check for negative and 0 income, the tax would result in 0 in that case.
Example for T2, we have an income worth 37k, we calculate the tax for the first 20k and to find the rest of the income we are using for T2 we substract the 20k to get the 17k left that will be used in the T2 tax calculations, the T1 and T2 then get added and that's the tax.
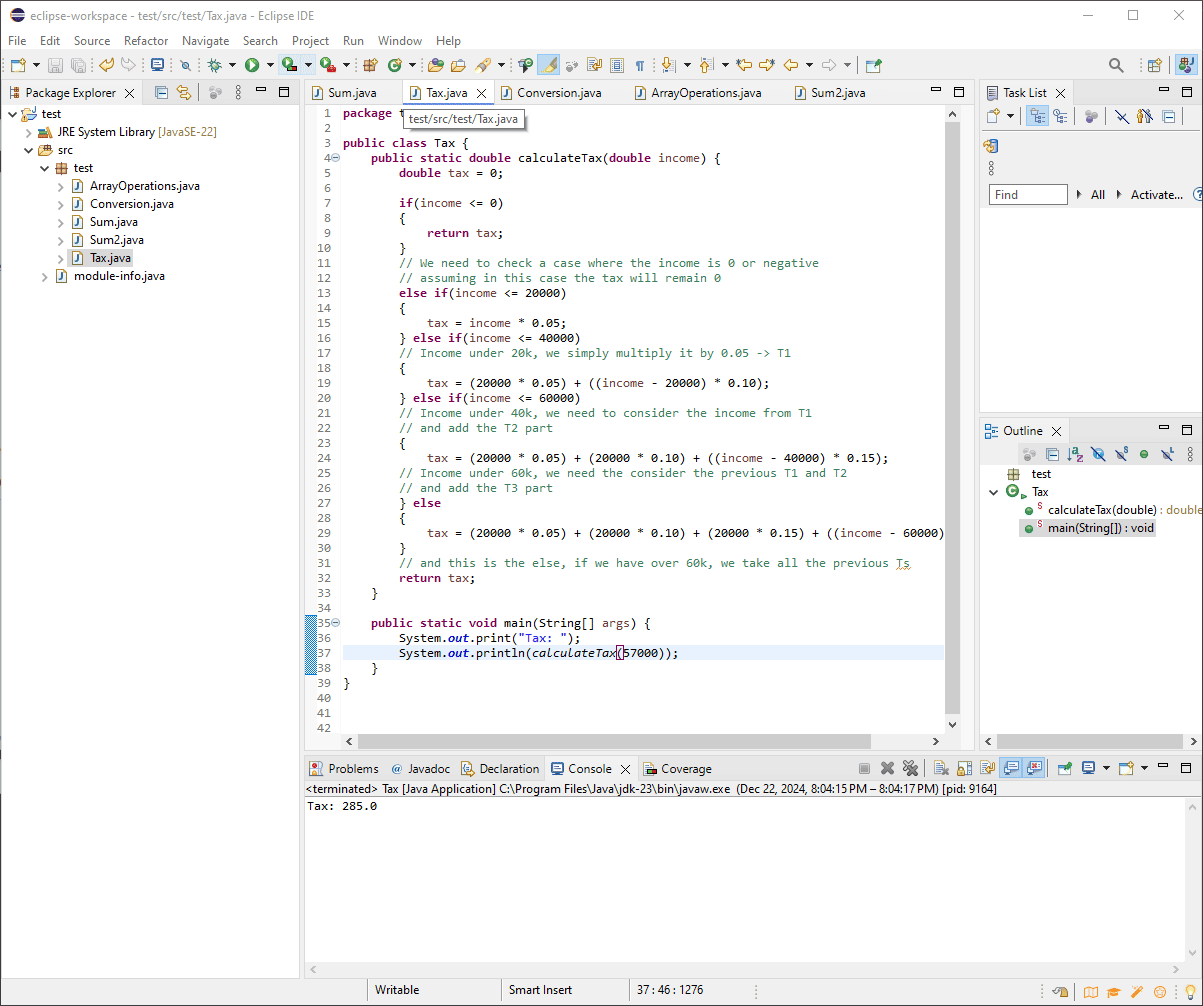
To get the result we simply call the function in the main and add the desired value as a parameter to the function.
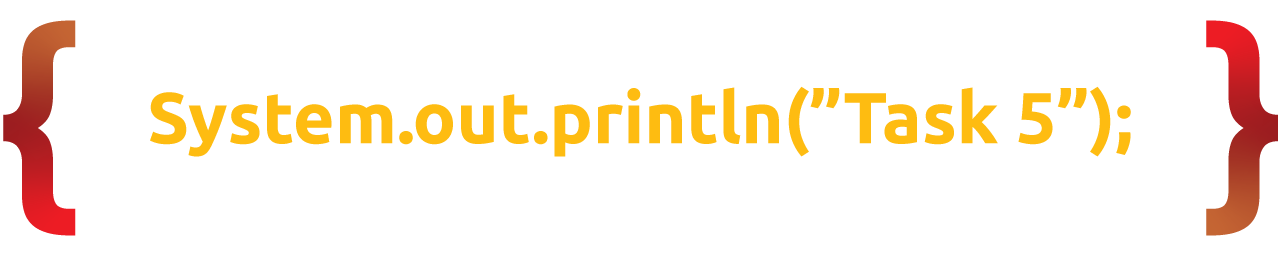
Using the same program structure as in the previous exercise, write a program named Conversion.java that takes a character c as a parameter and:
If the character is lowercase, convert it to uppercase and display the following message:
"The uppercase equivalent of c is ..."
If the character is uppercase, convert it to lowercase and display the following message:
"The lowercase equivalent of c is ..."
If the character is not a letter, display the following message:
"c is not a letter."
Here we are starting with getting an input from the user via scanner
, we prompt the user to write down a character. We store the character in the c
variable and add the .charAt(0)
in case the user drops more then one character in the input. If the user writes a string, we get the first character only.
Now with some simple if-statements we check if the character is either lower case or uppercase and change it accordingly, if it's not one of these two it's not a letter so we go to the else branch.
Below I will add a GIF with me running the code with different inputs, lower case, upper case, string, numbers.
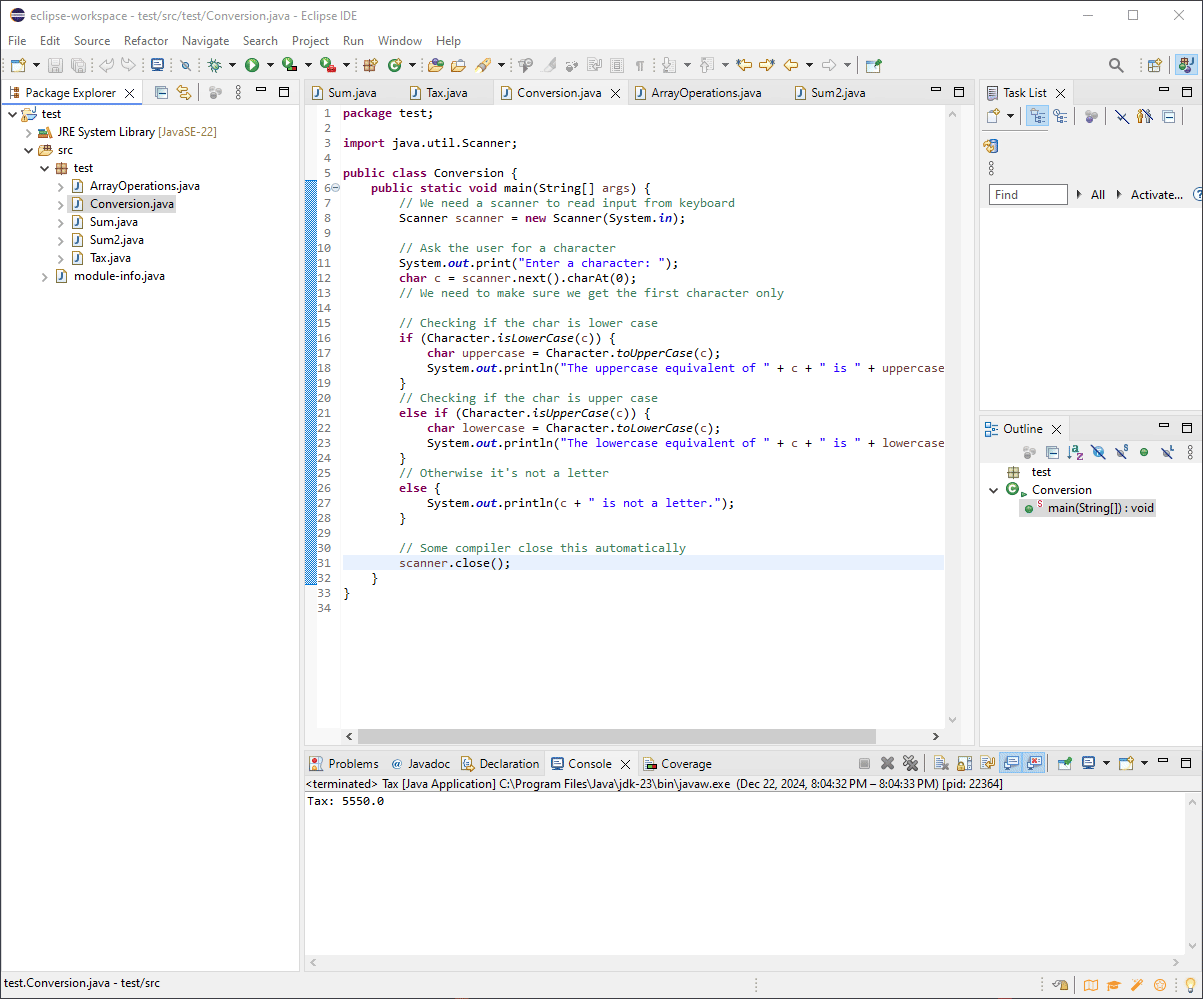
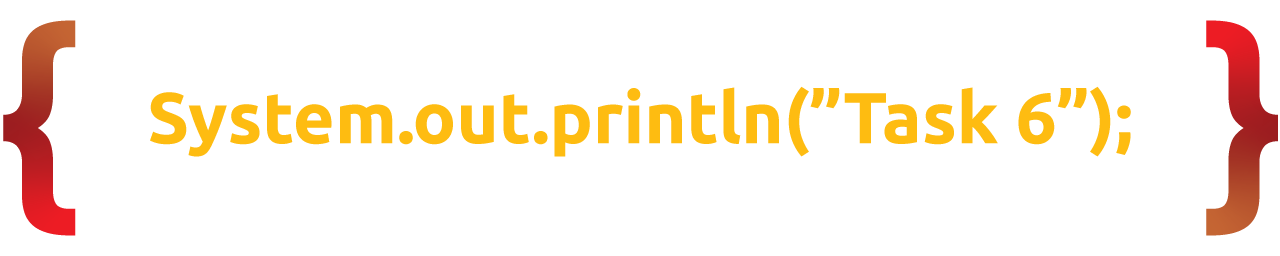
Using a similar structure as in the previous exercises, write a program named ArrayOperations.java that performs operations on an integer array. The program should:
Take an array of integers as input.
Provide the following options for operations:
- Find the Largest Element: Identify and display the largest element in the array.
Example Message:
"The largest element in the array is ..." - Calculate the Sum of All Elements: Compute and display the sum of all integers in the array.
Example Message:
"The sum of all elements in the array is ..." - Sort the Array in Ascending Order: Sort the elements and display the sorted array.
Example Message:
"The sorted array is: [ ... ]"
If the array is empty, display the following message:
"The array is empty. No operations can be performed."
We need to start by getting the size of the array, again we are using scanner
and store the size value in size
variable.
For point 3 we need to check if the array is empty, we can add that right at the start after getting the size input. We use the return
to stop the function here as the next part will not be needed if size
is 0.
size
is not 0 we move forward and read the array values using a loop. We have the array filled now, let's show the choices to our user. Using a While Loop with true (infinite loop), we show the text choices and scan the value from the user, with this scanned value in the choice
variable we move to a Switch Case statement where the choice
will be used to choose a case in our Switch, each case being a direct representative for the choices printed above for our user.
- case 1: largest number in array
- case 2: sum in the array
- case 3: sort of the array
- case 4: the exit from the switch - case
- default: an invalid number entered, user is asked to chose another option, a valid one
Everything is ready let's see the functions created for our cases. The largest value in the array, we assume the first element is the largest, we loop through the array (using an enhanced for loop, it's simplified and easier to understand), we find a value bigger than the current largest, if yes, the new largest value is the current element, and we move like this through the whole array, once done we return the largest element.
The next function is the sum one, we apply the same concept used a few tasks above where we added the first 100 integers, in that case we added the i
value, here we add the value under the i
position, we can't see the i
in the loop because we use an enhanced for loop to simplify things. Once done we return the sum.
And the last one, array sorting, we copy the array into a new one to be sorted, in this case it would not affect us to use the original array and do the sorting there, but if we'd need new choices later, some might require the original array sorting, sorting it might alter the result.
Once we have a copy of the original array, we sort it and return it.
Here's a GIF (30 seconds long) with the choices:
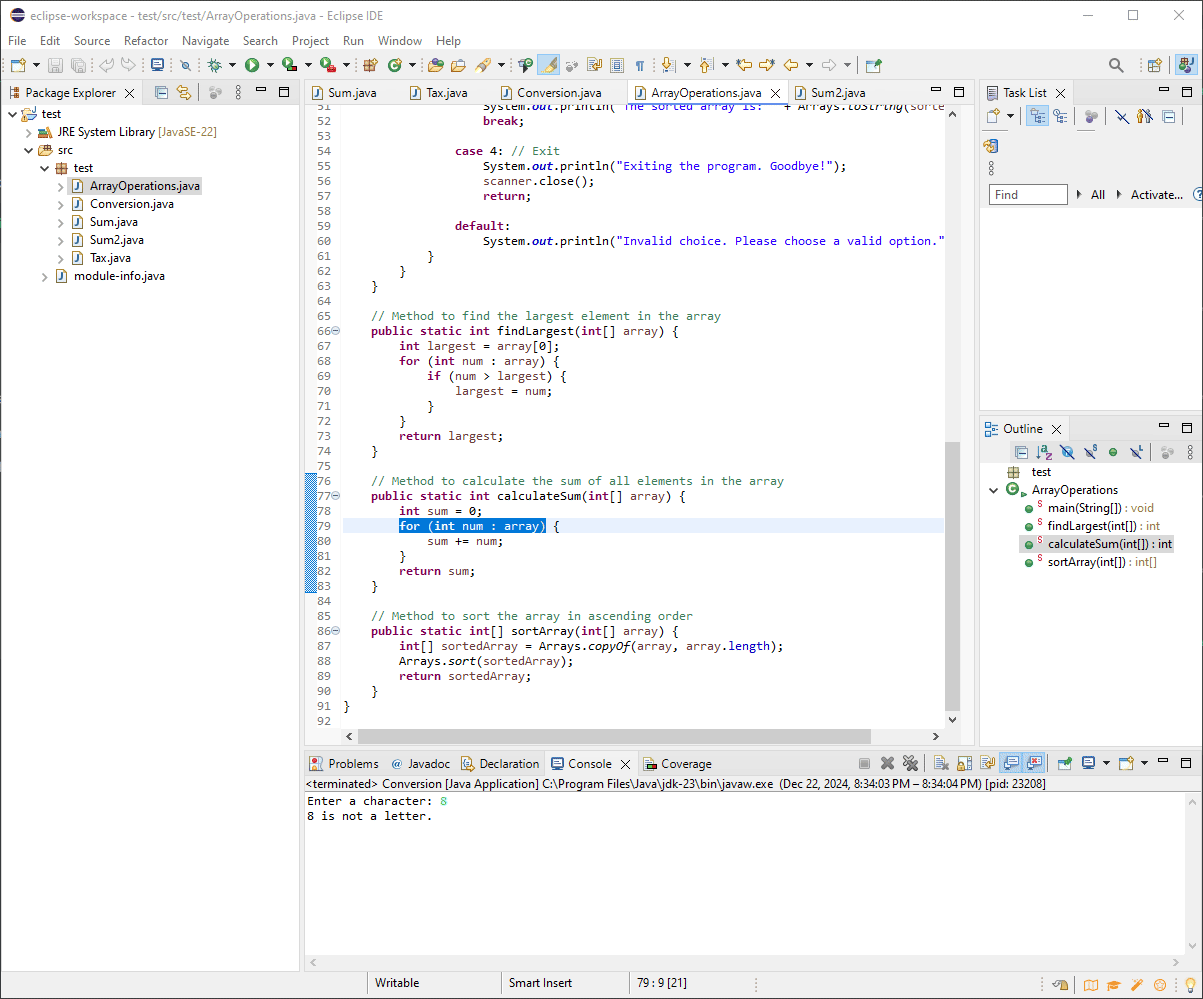
That was it for today's homework, in the end I would like to invite @titans, @mojociocio and @r0ssi to participate.
Can't wait for the next week's Java, thank you @kouba01 for this week's course.
Comments